Developing a Billing System in C++: Item Management and Invoice Generation
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings to you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
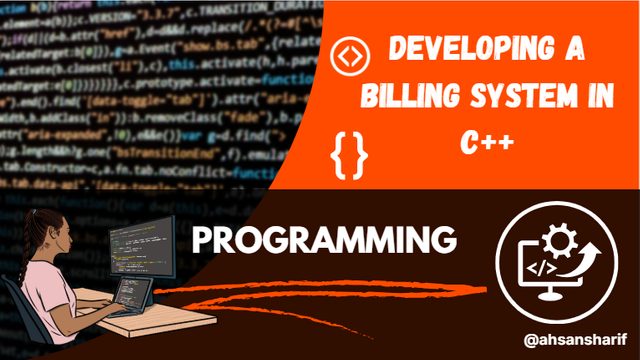
Canva Design
I hope everyone is well and enjoying their lives. Today I have come up with a mini project using C++ language in which there is a system which is a billing system. In this, any user will add his item. This system will collect it and then calculate its total. When it calculates the total according to its price, it generates an invoice if the user wants.
Structure:
From this, we will now look at the entire project step by step to see what is working in which place, so first of all, let's talk about the structure.
A structure called Item
has been used to define each product, which consists of three attributes. Its purpose is to store the name, price, or quantity of each item and then add it to the billing system.
C++ Code:

Menu Display Function:
Next to this is the function displayMenu()
that shows the user a menu in which the user can select these items and its purpose is that the user interacts with the system function, can add items there, can get their invoice prepared, or can exit the program.
C++ Code:

Add Items To The System:
Here, the addItem()
function allows the user to write about items, including their name, price, and quantity, and it stores them in the item
.
C++ Code:
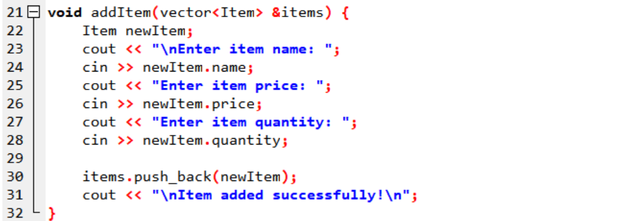
Here, first, the user will be asked to enter the name of the item, then the price of the item will be input, and then the quantity of the item will be input.
This item is added to the list using the
push_back()
function.
Here the function asks the user to add items one by one and then stores them in a vector of items
and keeps them in a track.
Generate Invoice:
The generateInvoice()
function calculates the price of all items and then displays the invoice. Apart from this, it also shows the total amount of all the items.
C++ Code:
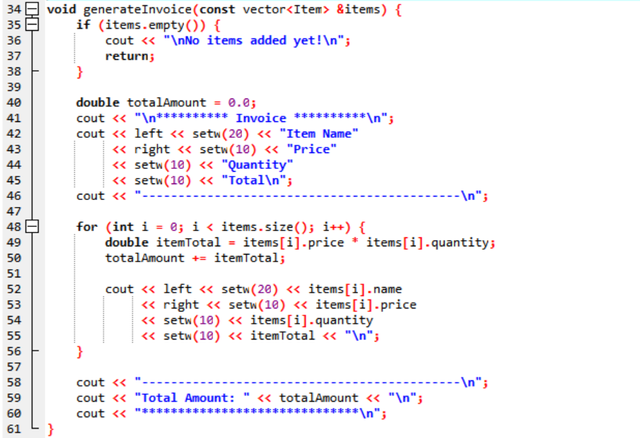
First, it will check if the item exists in the list. If the list is empty, it will display a message that no items have been added.
If an item is present in the list, then it will calculate its price. It calculates the price of the item by multiplying the quantity of an item.
This program mentions the total price of each item above the overall bill.
Then it prints the invoice, which has the name, quantity, price written on it, and the total price at the end.
Finally, it combines the total price of all items and displays the amount the user is paying.
The final purpose of this function is to generate a detailed invoice that shows the total bill by adding up the prices and quantities of all the items purchased by the user.
Main Program:
In our program, this main()
function controls the entire program. It keeps the user displayed all these menus and takes action accordingly, according to the user's choice.
C++ Code:
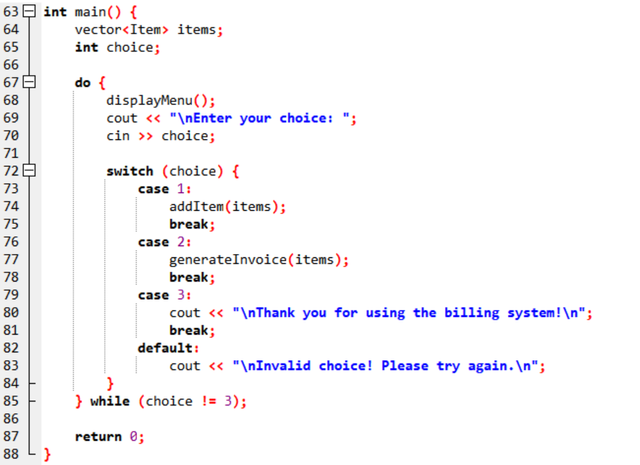
Menus are shown to the user using the displayMenu()
.
The user has three options here. In the first option, if he wants to add an item, it will be added through addItem()
. In the second function, if he wants to generate an invoice, after adding the item, the generateInvoice()
function is used and it calculates the total all. In the third option, there is an exit option where the user can exit the program if he has no other work to do.
The purpose of this main()
function is that it is connected to all functions and it adds items generates their invoices or removes them from the program based on what the user is interacting with.
Working:
In the working of this project, I am showing you in a video below how it works. In this video, you can see that we have three options. We have to choose one of the three. If we want to add items, we will use the first option. If we have added an item, then we will generate its invoice. For this, we will choose the second option. And the third option is to exit our program if our work is complete. Apart from this, if you choose any option, it will show the invalid.
Final Code:
#include <iostream>
#include <iomanip>
#include <vector>
#include <string>
using namespace std;
struct Item {
string name;
double price;
int quantity;
};
void displayMenu() {
cout << "\n*** Welcome to Store Billing System ***\n";
cout << "1. Add Item\n";
cout << "2. Generate Invoice\n";
cout << "3. Exit\n";
}
void addItem(vector<Item> &items) {
Item newItem;
cout << "\nEnter item name: ";
cin >> newItem.name;
cout << "Enter item price: ";
cin >> newItem.price;
cout << "Enter item quantity: ";
cin >> newItem.quantity;
items.push_back(newItem);
cout << "\nItem added successfully!\n";
}
void generateInvoice(const vector<Item> &items) {
if (items.empty()) {
cout << "\nNo items added yet!\n";
return;
}
double totalAmount = 0.0;
cout << "\n********** Invoice **********\n";
cout << left << setw(20) << "Item Name"
<< right << setw(10) << "Price"
<< setw(10) << "Quantity"
<< setw(10) << "Total\n";
cout << "--------------------------------------------\n";
for (int i = 0; i < items.size(); i++) {
double itemTotal = items[i].price * items[i].quantity;
totalAmount += itemTotal;
cout << left << setw(20) << items[i].name
<< right << setw(10) << items[i].price
<< setw(10) << items[i].quantity
<< setw(10) << itemTotal << "\n";
}
cout << "--------------------------------------------\n";
cout << "Total Amount: " << totalAmount << "\n";
cout << "******************************\n";
}
int main() {
vector<Item> items;
int choice;
do {
displayMenu();
cout << "\nEnter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addItem(items);
break;
case 2:
generateInvoice(items);
break;
case 3:
cout << "\nThank you for using the billing system!\n";
break;
default:
cout << "\nInvalid choice! Please try again.\n";
}
} while (choice != 3);
return 0;
}
This is the final coding of our project. I hope you all liked my project. Thank you all very much for stopping by. I would like to invite my friends @kouba01, @syedbaider, and @josepha to join this club.
Cc:
@kafio @mohammadfaisal @alejos7ven
I see that you’ve made the functional console-base billing system, but it lacks user friendly interface. Since I have already worked on this project in my practice session, I strongly recommend you to focusing on GUI next. Consider using the framework like Qt and other option is SFML in C++ to present clear input fields, buttons, and a professional layout. I review your code structure, you can separate your logic from the UI so each part remain more maintainable. It is also good practice to validate inputs. for example, prevent negative prices or quantities. But It is amazing project to improve the coding skillls and make improvements, keep it, I appretiate your efforts.
Thanks for your suggestions. The Qt framework is not installed on my laptop, which is why I missed the Kouba01 season because they had worked on Qt design. I tried very hard to install it on my Laptop, but I could not succeed, so I am using this kind of interface.