SLC21 Week2: Programmin arrays
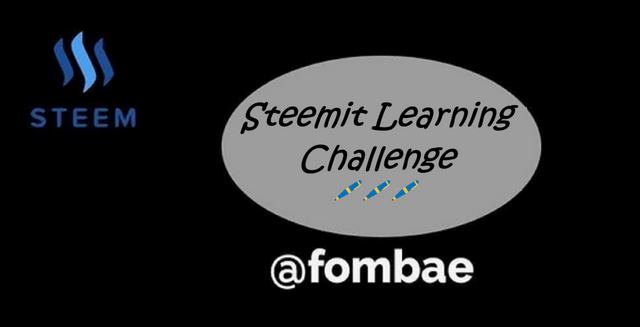
Greetings Steemit friends
Declare an array of any type, explain how to use an array, how to access array elements.
The array is like a container that holds identical data types. Do we have an array? We need to declare the array, assign value, and finally how have access to the data stored in the array.
Declare an array of integers (data type) that will hold 10 values by giving it a name. Arrays are made up of an index and an element. To have access to the element of the array, we will need the index. In my case, it will run from 0 to 9. Here we will have the data type and the array name.
Int serialNumber[10]
Assigning an element to each index is the next step when preparing an array to be used. I mentioned I would be working with an array holding 10 elements.
serialNumber[0] = 9521;
serialNumber[1] = 3761;
serialNumber[2] = 4521;
serialNumber[3] = 7321;
serialNumber[4] = 2521;
serialNumber[5] = 6421;
serialNumber[6] = 1521;
serialNumber[7] = 4528;
serialNumber[8] = 4825;
serialNumber[9] = 2587;
Finally, to access the array elements, we need the index for each element. Elements are identified by their index.
cout << "Element at index 0: " << serialNumber[0] << endl;
cout << "Element at index 1: " << serialNumber[1] << endl;
cout << "Element at index 2: " << serialNumber[2] << endl;
cout << "Element at index 3: " << serialNumber[3] << endl;
cout << "Element at index 4: " << serialNumber[4] << endl;;
cout << "Element at index 5: " << serialNumber[5] << endl;
cout << "Element at index 6: " << serialNumber[6] << endl;
cout << "Element at index 7: " << serialNumber[7] << endl;
cout << "Element at index 8: " << serialNumber[8] << endl;
cout << "Element at index 9: " << serialNumber[9] << endl;
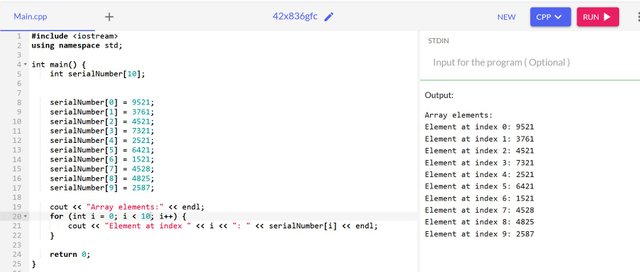
Advantages of an array over ordinary variables
Array helps reduce lines of code, making it easy to read and manipulate, as compared to variables which have to detail each variable for each value or element.
Arrays can be easily used to perform operations such as sorting, searching, etc. As compared to variables it is not possible to carry out such an operation with a number of variables.
Arrays can hold multiple elements of the same data type within a single variable while it will have to declare multiple variables to achieve this.
Arrays make use of indexes, which make it easy to loop through large data. With indices, it is easy to pick a particular element and perform an operation.
Array helps save coding time, as you just have to use a single viable index and not waste time typing different variables when performing an operation.
Arrays make it easy to pass data to a function. With one variable you can pass all necessary elements needed in a particular function.
What is the name of the array? What will happen if you display this value on the screen? What does cout<<a+2;that mean cout<<a-2;? If cout<<a;4,000 is displayed when outputting, then how much will it bea+1?
The name of the array can be seen as the address memory of the elements. The array name is the container holding the elements and can be used to point to a particular element by using the index.
In my previous task, I used serialNumber as the name of the array. So the array serialNumber
points to the elements using the index. In the first case, serialNumber
will point to serialNumber[0]
to get the first element.
So when we display it on the screen, it will display a memory of the first address following the location of the memory.
- What does
cout << a + 2;
mean?
a
hear is the array name which moves with the pointer a[0]
. The + sign means adding 2, which means moving the point forward by 2. So our new memory location will be a[2]
holding a new pointer. cout << a + 2; has moved from cout << a[0] + 2
to cout << a[2]
.
Here now, the element displaced will be the value of a[2]
.
- What does
cout << a - 2;
mean?
Okay, here we have to move backward from the point where the array starts a[0]
. This is practically not possible and we will be trying to get an element out of the array. Following the operation, it will mean we are trying to display a[-2]
value. This memory doesn't exist and can lead to an unusual behavior if applied to our program.
- If
cout << a;
displays 4000 as output, then how much willcout << a + 1;
be?
If cout << a;
displays 4000, also means a[0] = 4000
. 4000 is the value assigned to the first index of the array a
. cout << a + 1
, means performing an arithmetical operation which will then move from a[0]
to a[1]
.
Given that our array is of data type integer, cout << a + 1; 4001
.
Can an array have two dimensions?
A two-dimensional Array is an array with an element having two indices (row and column). It is like picking an element from a table and making use of a specific row and column.
Here we have to specify the number of rows and columns when declaring the array. Just like any other array:
- Declare array
With a Two-Dimensional Array, we have a main curly braces and each row in a respective curly braces.
int numbers[3][4];
- Access element.
Here, we make use of the indices (row and column) to have access to the elements in a two-dimensional array. The first index represents the row, while the second represents the column.
In case we want to have access to all the elements in the array. Just as we used a loop for the case of a normal array, we will have to use a nested loop for two-dimensional arrays.
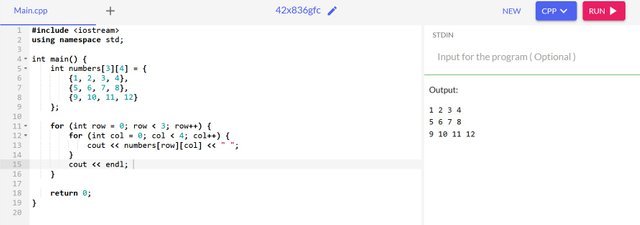
Two-dimensional arrays are used in cases where we want to hold data in a table format, making it easy to sort and manipulate.
Write a random number in the variable
k. int k=rand()101rand()101rand()101+500;
Try to solve the task of finding divisors from the last lesson more efficiently**
Here we start by generating the random number using the variable k.
int k=rand()101rand()101rand()101+500;
For my array to contain numbers from 0 to 100, I will update the variable to look like this.
int k = rand() % 101 * rand() % 101 * rand() % 101 + 500;
This will give the product of three random numbers plus 500.
In the next step, there is no need to do any checks like in the previous task. We will just go ahead to find the square root of the variable k and get its divisor.
Finally, each output is stored in an array which can be displayed using a loop.
int divi_store[500];
declare array for divisors, which can hold elements between 0 to 999.
int divi_count = 0;
Will be used to generate the index
Find divisors efficiently up to the square root of k, which is a random number.
The divisors are stored in the 'divi_store' array, while the divi_count holds the number of elements in the divi_store array.
Now we display the divi_store which is stored in the array using the for loop using the divi_cout as indexes.
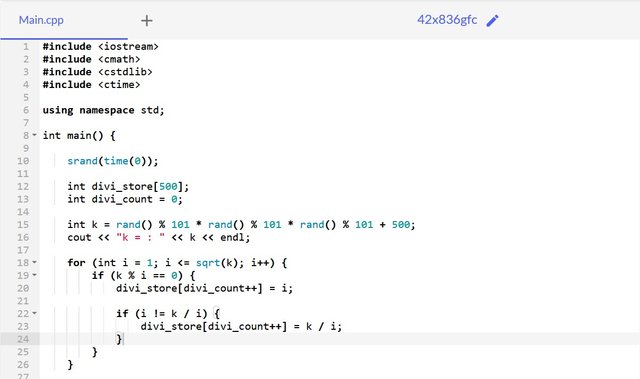
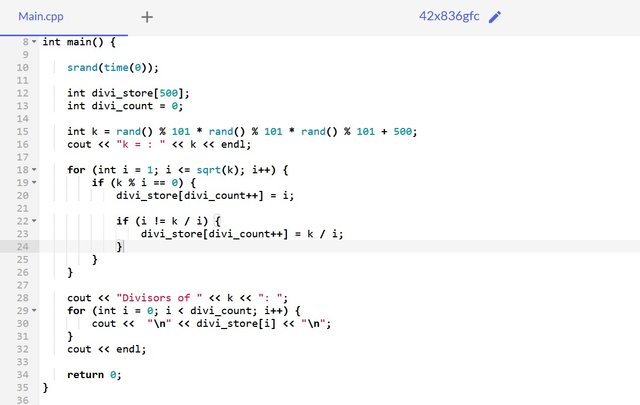
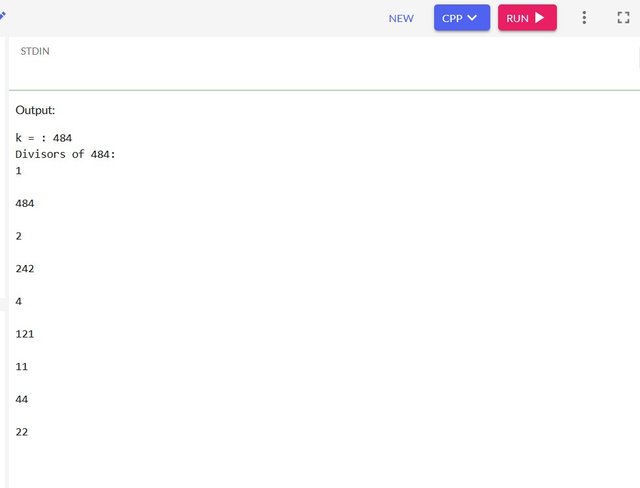
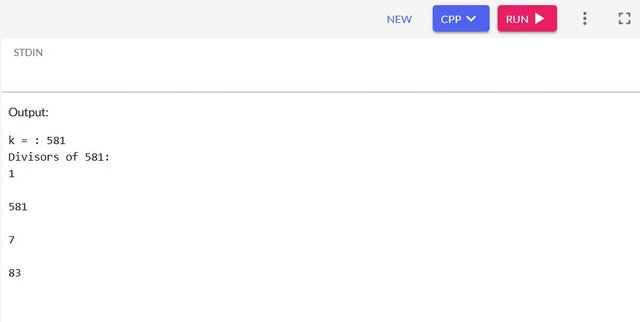
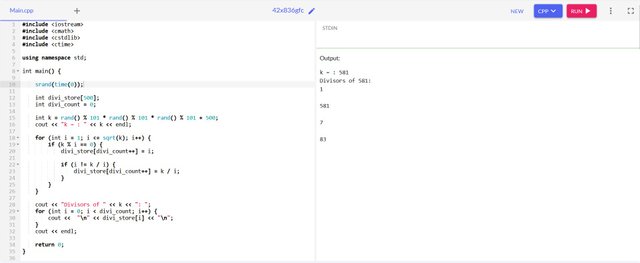
Note we are storing only divisors in the array, that is why we have the index skipping when displayed.
Fill the array with 55 numbers with random numbers from 10 to 50. If there is a number 37 among the elements of the array, print it yes, and if there is no such number, print it no
Here we will start by declaring a variable and the array of numbers.
int SIZE = 55;
int numbers[55];
Next will fill the elements with random numbers within 10 and 50. For us to be able to cover the range, we need to divide by 41.
50 -10 + 1 = 41
Rand()% 41 will give a number with the range from 0 to 49, but the task required from 10 to 50. So by adding 10, we will shift the range from 10 to 50
formula rand() % 41 + 10
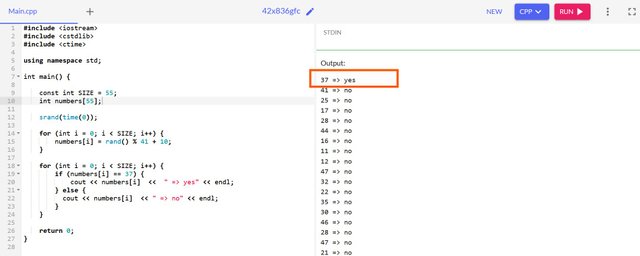
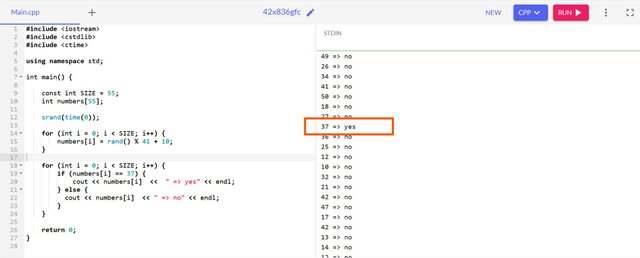
Now, I will go ahead to check for the number 37 if it is found among the elements. Then go ahead and print yes, and the rest print no.
Fill an array of 66 numbers with random numbers from 12 to 60. Replace even elements with 7 and odd elements with 77
Here the beginning will not be different from the above task, only the operation will change as we proceed.
Here we will start by declaring variables and the array of numbers.
int SIZE = 66;
int numbers[66];
Next will fill the elements with random numbers within 10 and 50. For us to be able to cover the range, we need to divide by 41.
60 -12 + 1 = 49
Rand() % 49 will give a number with the range from 0 to 48, but the task required from 12 to 60. So by adding 12, we will shift the range from 12 to 60.
formula rand() % 49 + 12
Now, within the loop. We check for even numbers and replace them with 7, while odd numbers will be replaced with 77. For every event element, a new value is set which is 7. The same goes for odd elements, where a new value is set which is 77. At this point, the new values override the previous values set in the array.
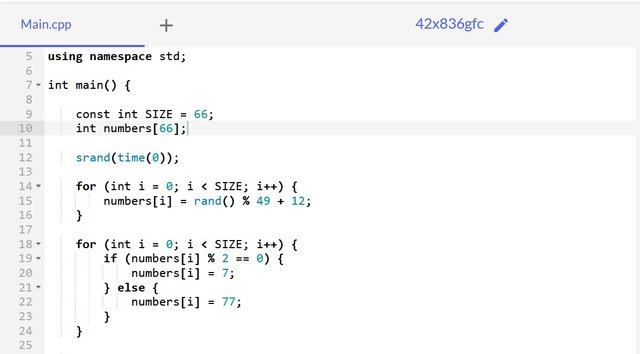
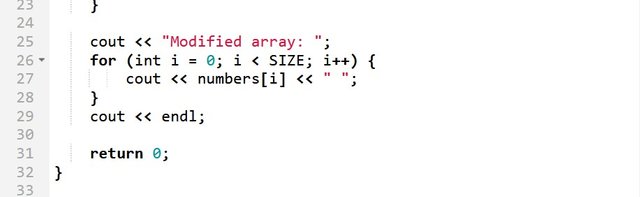
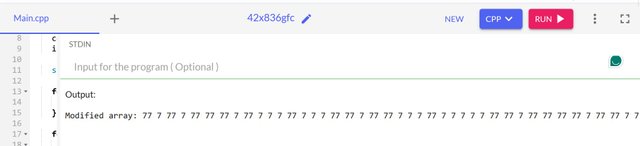
Fill the array of 77 numbers with random numbers from 102 to 707. Find the two largest numbers.
Here we are not different from the above tasks will use the same steps to have the random number within the range from 102 to 707
Here we will start by declaring a variable and the array of numbers. INT_MIN is set to a variable because it is an initial value when searching for the largest number in an array or data set.
int SIZE = 77;
int numbers[77];
int largest = INT_MIN;
int secondLargest = INT_MIN;
Next will fill the elements with random numbers within 102 and 707. For us to be able to cover the range, we need to divide by 606.
707 -102 + 1 = 606
Rand() % 606 will give numbers with the range from 0 to 605, but the task required from 102 to 707. So by adding 102, we will shift the range from 102 to 707.
formula rand() % 606 + 102
Next, is to get the "the two largest numbers". To have a more clear understanding, it will be possible to get the two distinct largest numbers. That way, if a large number appears more than once. Only one will be picked and the next large number will be unique and different from the initial large number.
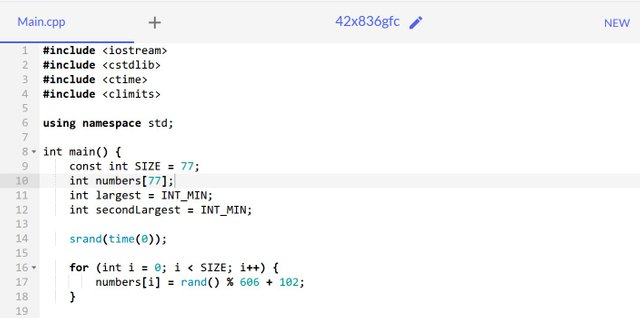
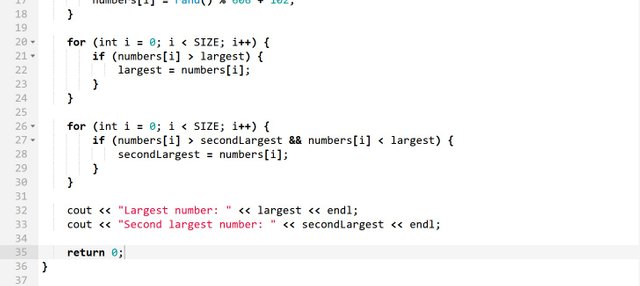
After generating the 77 random numbers within the range of 102 to 707. next will loop through to get the largest number. The large number is stored in a variable and another loop is initiated. This time a check is done to avoid the larger number, so as to get the second large number.
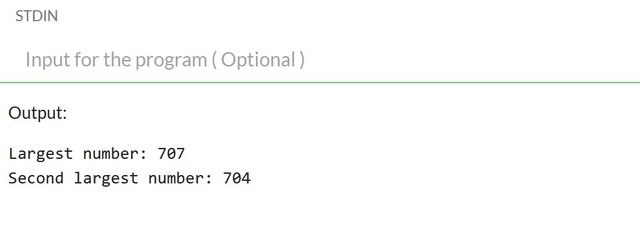
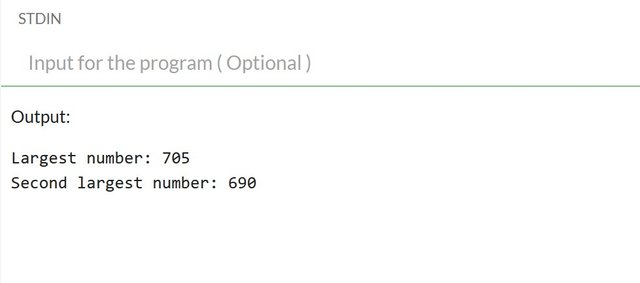
Here we can get the two large numbers that are different from each other.
Cheers
Thanks for dropping by
@fombae