SLC21 Week2 - Programming arrays
Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 21 week 1 by @sergeyk under the umbrella of steemit team. It is about programming arrays. Let us start exploring this week's teaching course.
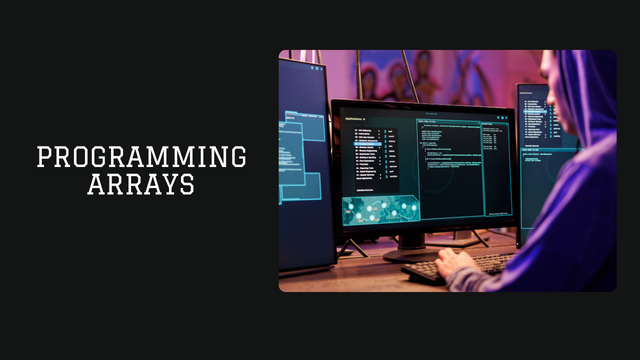.png)
I will use the C++ language to perform all the tasks.
explain how to use an array, how to access array elements.
In C++ we can declare an array by giving the data type, the name of the array and the number of the elements which it can hold.
Here is an array with the data type integers with the 5 elements.
int numbers[5];
This creates an array of numbers
. It can hold 5 integers. The elements will garbage values until we initialize them.
Assigning Values to Array Elements
We can assign values to the elements in the array with the help of the index. The important thing here is that the index starts from 0. Here is the example:
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
numbers[4] = 5;
We can also initialize the array at the time of the declaration.
int numbers[5] = {1, 2, 3, 4, 5};
Accessing Array Elements
In order to access the array elements we can use their index. Each index holds a value. If we want to access the value in the array we need to access the index and the index will return that value.
int firstElement = numbers[0];
int thirdElement = numbers[2];
cout << "First element: " << firstElement << endl; // Displays 1
cout << "Third element: " << thirdElement << endl; // Displays 3
Displaying Array Elements
We can use a loop to display the elements in the array. Here I am using a for loop to display the elements of the array.
for (int i = 0; i < 5; i++) {
cout << "Element at index " << i << ": " << numbers[i] << endl;
}
This will display all the values in the numbers
array.
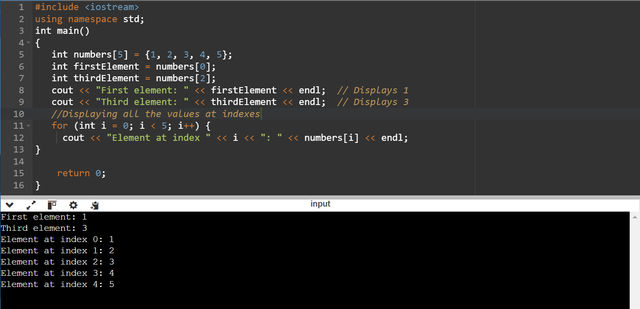
Advantages of Arrays over Ordinary Variables
Here are the advantages of the array over the ordinary numbers:
Efficient Storage: An array can store multiple values under one name, making it more memory-efficient than declaring separate variables for each item.
Easy Access: We can easily access any element in the array with the help of the index.
Manageability: We can manage related data easily with arrays such as looping through values compared to individual variables.
Memory Management: Arrays are stored in the contiguous memory locations. This makes them more efficient to manage the memory access and the use of memory.
Arrays are powerful features in any programming languages. When we need to work with a list of items we can access and manage that list of items as a single unit.
What is the name of the array? What will happen if you display this value on the screen? What does cout<<a+2;that mean cout<<a-2;? If cout<<a;4,000 is displayed when outputting, then how much will it bea+1?
In C++ the name of the array indicates a pointer to the first of the array. For example: a
in int a[5];
. When we output the name of the array using cout << a;
then it will display the memory address of the first element in the array. It will not display the content of the array.
Explanation
I am breaking down the questions one by one:
What is the name of the array?
- The array name
a
(inint a[5];
) is a pointer to the first element of the array. So,a
points to the address ofa[0]
.
- The array name
What happens if you display this value on the screen?
What does
cout << a + 2;
mean?a + 2
performs pointer arithmetic. Asa
is a pointer to the start of the arraya + 2
points to the memory address of the third element such asa[2]
. When we outputcout << a + 2;
, it will display the address ofa[2]
not its value.
- Similarly
a - 2
is not meaningful in this context as it tries to access an address before the start of the array because the array is starting froma
.
- If
cout << a;
displays4000
, then what willa + 1
display?- If the address of
a[0]
is4000
, thena + 1
will display4004
by assumingint
takes up 4 bytes. In C++, pointer arithmetic takes the data type size into account so adding1
to a pointer of typeint
moves 4 bytes forward.
- If the address of
Can an array have two dimensions?
Yes an array can have two dimensions. A two dimensional array can be thought of as an array of arrays. It is often used to represent data in a matrix or table format.
Declaring a Two Dimensional Array
In C++ we can declare a 2D array by specifying two dimensions. In the two dimensions the number of rows and the number of columns are specified. For example:
int matrix[3][4];
It will create a 2D array with the name matrix
which has 3 rows and 4 columns. Each element in this array can be accessed by using two indices. One indice is used for the row and the other is used to represent the column.
Initializing a Two Dimensional Array
We can initialize a 2D array at the time of it's declaration such as:
int matrix[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
This will create a 3x4 matrix with the specified values.
Accessing Elements in a Two Dimensional Array
Inorder to access and modify the elements we use row and column indices. For example:
matrix[0][1] = 20; // Sets the element in the first row, second column to 20
int value = matrix[2][3]; // Accesses the element in the third row, fourth column
Displaying a Two Dimensional Array
In order to display a 2D array we use nested loops:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
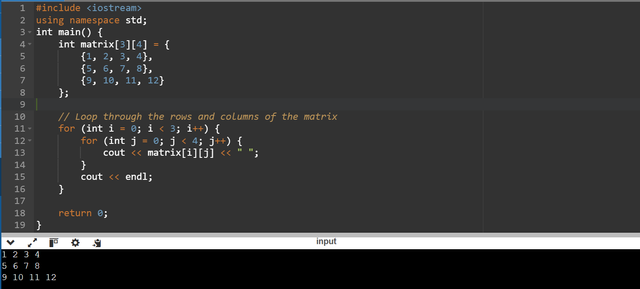
Advantages of Two Dimensional Arrays
2D arrays are useful when we deal with the grid like structures such as:
- Matrices for mathematical computations.
- Tables to store data in rows and columns.
- Grids in applications like games or simulations.
Two dimensional arrays provide a structured way to handle data that has both rows and columns. It makes it easier to organise and process information in a logical format.
Write a random number in the variable k
To improve the efficiency of finding divisors of a number k
and storing them in an array we can reduce the number of iterations by only checking up to the square root of k
. For each divisor found below the square root we can directly determine its paired divisor above the square root. In this way we only check up to sqrt(k)
to make the algorithm much faster.
Here is how we can achieve this:
- Generate the Random Number
k
as specified. - Find Divisors Efficiently by iterating only up to
sqrt(k)
. - Store Divisors in an Array.
Here is the code in C++:
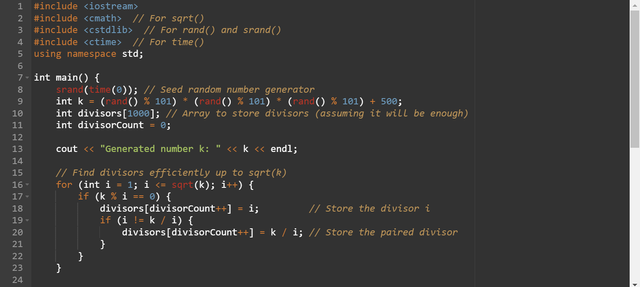
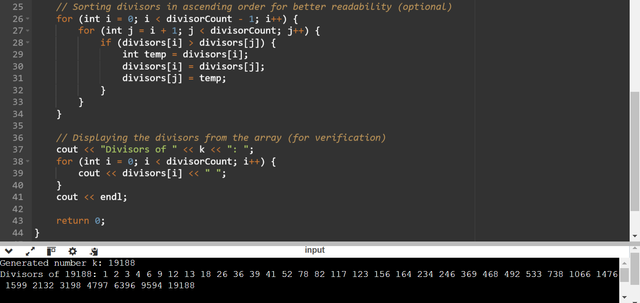
After running the code again it is an another example with a random number.

Explanation
- Random Number Generation:
k
is generated based on the specification. It is a large random integer that is calculated usingrand()
values. - Efficient Divisor Calculation:
- We loop only up to
sqrt(k)
to check ifi
dividesk
. - If
i
is a divisor such ask % i == 0
theni
andk / i
are both divisors ofk
. - This approach saves time by minimizing the number of iterations especially for large values of
k
.
- We loop only up to
- Array Storage: Divisors are stored in the array
divisors
anddivisorCount
keeps track of the number of divisors found. Each time a divisor is found it is incremented. - Sorting: I have written this code to sort the divisors in ascending order so that they appear from smallest to largest in an aligned way.
This code finds and stores all divisors of k
efficiently in the array divisors
without using a function for array filling.
Fill the array with 55 numbers with random numbers from 10 to 50. If there is a number 37 among the elements of the array, print it yes, and if there is no such number, print itno
We can fill an array with 55 random numbers between 10 and 50 then we will check if the number 37 is among them. If the number 37 is found then the program will print "yes"
and this number is not found it will print "no"
.
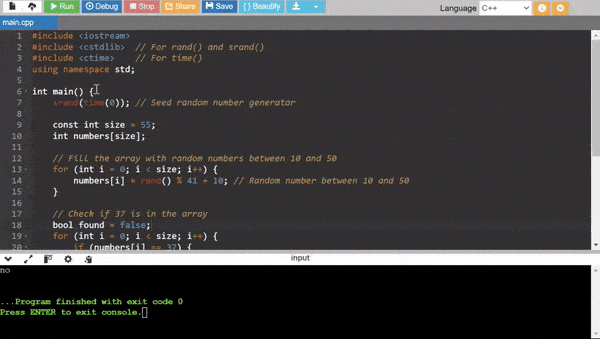
Explanation
Random Number Generation:
rand() % 41 + 10
generates random numbers between 10 and 50:rand() % 41
generates a random number from 0 to 40.- Adding 10 shifts this range to 10 to 50.
Checking for the Number 37:
- A loop iterates through the array to check if any element in the array is equal to 37.
- If 37 is found then
found
is set totrue
and the loop stops.
Printing the Result:
- If
found
istrue
then it prints"yes"
otherwise it prints"no"
.
- If
This code accomplishes the task efficiently by filling the array and checking for 37 in a single pass.
Fill an array of 66 numbers with random numbers from 12 to 60. Replace even elements with 7 and odd elements with 77
Here is the method that we can fill an array with 66 random numbers between 12 and 60. Then this method will replace even elements with 7 and all odd elements with 77.
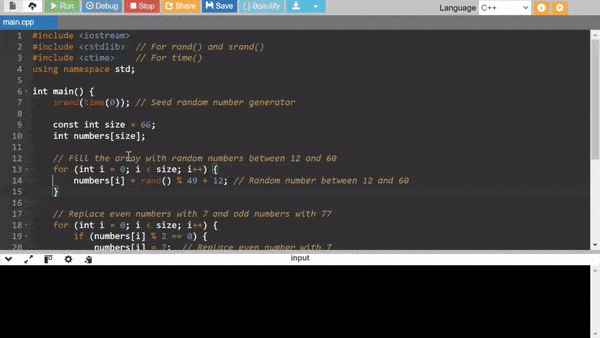
Explanation
Random Number Generation:
rand() % 49 + 12
generates random numbers from 12 to 60:rand() % 49
generates a random number from 0 to 48.- Adding 12 shifts this range to 12 to 60.
Replacing Elements:
- A loop iterates over each element in the array.
- If the element is even (
numbers[i] % 2 == 0
) then it is replaced with7
. - If the element is odd then it is replaced with
77
.
Displaying the Modified Array:
- A loop displays the modified array to show the results after replacing the values.
This code fills the array and modifies it. After that it prints the final version of the array with all even numbers replaced by 7
and odd numbers replaced by 77
.
Fill the array of 77 numbers with random numbers from 102 to 707. Find the two largest numbers. But the phrase "the two largest numbers" can have many interpretations. Therefore, first explain well how this phrase was understood. And then solve the problem.
The phrase "the two largest numbers" can indeed have multiple interpretations. Here are some possible ways to interpret it and the approach that we will take:
Two Distinct Largest Values: The goal would be to find the two largest distinct numbers in the array. For instance, if the array contains
707, 707, 600, 500
, the two largest distinct values are707
and600
.Top Two Values, Allowing Duplicates: This approach simply takes the two highest numbers in the array, regardless of whether they are the same. For example, if the array is
707, 707, 600, 500
, the two largest numbers would be707
and707
.
For this solution, we’ll assume the first interpretation: finding two distinct largest values in the array. If the array contains duplicate values, the second largest distinct number will be returned.
Here's how we can accomplish this in C++:
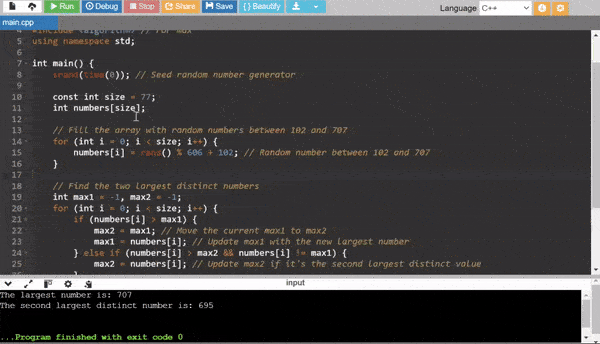
Explanation:
Random Number Generation:
rand() % 606 + 102
generates random numbers between 102 and 707:rand() % 606
gives a range from 0 to 605.- Adding 102 shifts this range to 102 to 707.
Finding the Two Largest Distinct Values:
- We initialize
max1
andmax2
to-1
to represent that no values have been assigned yet. - We iterate through the array:
- If
numbers[i]
is greater thanmax1
, we updatemax2
to hold the previousmax1
value, then setmax1
to the new highest number. - If
numbers[i]
is betweenmax1
andmax2
(and not equal tomax1
), we updatemax2
.
- If
- We initialize
Output:
max1
andmax2
will now hold the two largest distinct values in the array. We print them to verify the results.
This method ensures we find the two largest distinct numbers efficiently in a single pass through the array.
I invite @wirngo, @suryati1, and @chant to join this contest.
I have compiled all the code of the tasks in a famous online compiler which is OnlineGDB.
Upvoted! Thank you for supporting witness @jswit.
Terimakasih teman sudah mengundang saya di kontes yang sangat luar biasa ini, semoga anda beruntung ya 🥰
Thank you dear
Thank you dear
Sama-sama 🤗🌹
Hi @sergeyk are you here? My post is 3 days old. You have checked the posts which were published after mine. I think you forget.