C# Tutorial 006: Lists & Arrays
Description
In this tutorial I will show you how to creates lists and arrays and how to get the values within them. I will also show explain the difference between the 2 and when to use which one. The tutorial is meant for beginning programmers meaning it will only cover the very basics and keep the explanation and examples simple.Used program
Visual Studio 2015 – C# console applicationMaterial
This tutorial will teach you the following:• Creating and using a list
• Creating and using an array
Creating and using a list
A list in C# can store multiple values of a certain type, so when creating a list you must also declare of which type the list will be. Creating a list is slightly different from creating an integer or double, because you will first have to initialize the list (creating it) and only then you can add values to the list. Because you can continuously add values to a list after creating it the list does not have a fixed size (resizable), making it different from an array. In order to demonstrate how to create a list and add values to it I will create a list that adds each (string) input of the user to it.
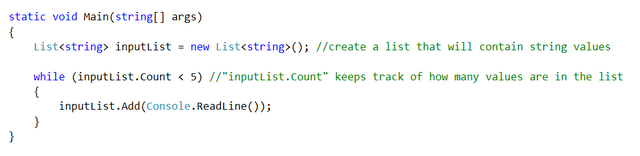
There are really only 2 steps to adding data to a list, but getting data can be a bit trickier. You can get a value of a list by calling one of the positions (the first position starts at 0!), but if you want to get a specific value from the list you will have to loop through the list to find it. See the example for both ways to get data from a list.
Please keep in mind that when getting the “Count” of a list, it will return a value that is 1 higher than the last position in the list. This is because the counting in a list starts at 0, while the counting of an amount of things starts at 1.
Creating and using an array
An array is quite similar in its purpose as a list. Just like the list you can store values in it of a certain type and getting the values from the array is also very similar to how you’d do it with a list. The main difference though is that an array cannot increase or decrease in size after it is created, it is not resizable. You will want to create an array instead of a list when you know the size will never change after its initial creation. I will now create the same program as I created when I demonstrated a list in order to demonstrate how to create and use an array. I will also show how to make a multidimensional array, which is used the same as a normal array apart from how you create it.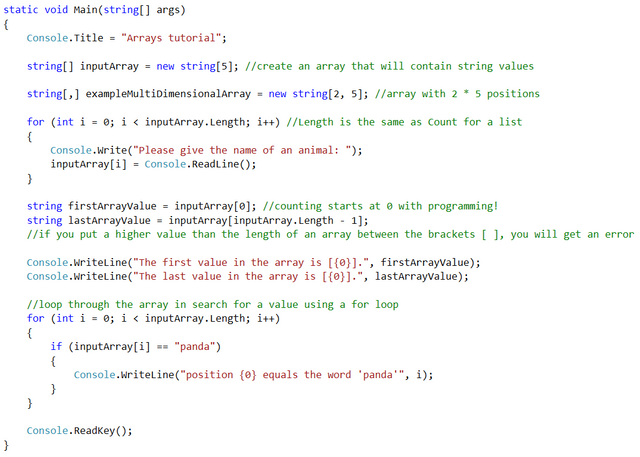
Series is doing well. Might be worth doing a follow up on the use cases for the different types Enumerable, Array, List, Dictionary etc. What they are good at and and when they are slow. Diction lookup vs array scan etc
Thanks for the feedback! I was personally thinking to first teach a bunch of the basics before moving on to Enumerable and Dictionary,
I'm also not really a big fan of using Dictionary after I ran into some trouble with it when developing a game on Unity.
What was the problem you had with Dictionary?
I use them a fair deal in my code but do not tend to pass them around. I prefer to pass their lookup function around. So if I have a Dictionary<int, Person> I will pass around a Func<int, Person>
That way I do not care what the storage is :)
Unity is a different space as threaded, the C# collections are not thread safe
The XmlSerializer I had to use could not serialize Dictionaries. At first I had a workaround, but it just became too much of a struggle with several dictionaries so I had to switch To Lists or Arrays.
Btw, check out my posts. I have a series on pushing C# outside the OO norms