PeakeCoin Matic Contract
I have been held up trying to figure out a way to bridge the gaps and make the token more usable or have a higher use case.
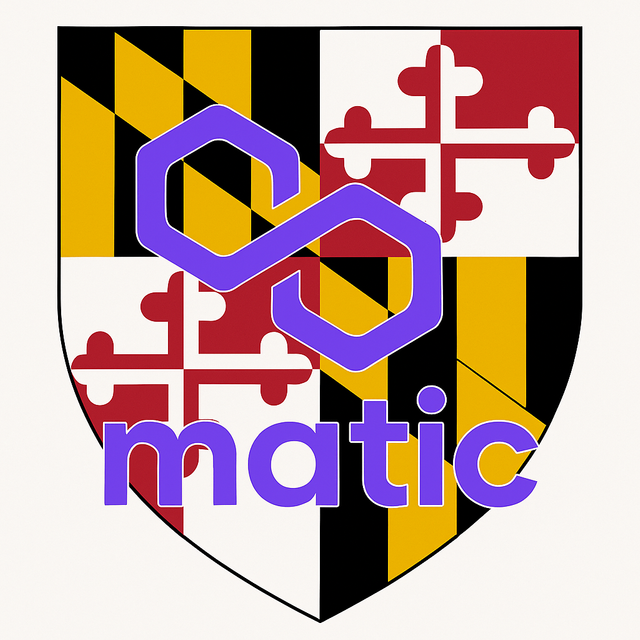
I took some time out to begin writing the Matic Smart Contract for PEK.
The amount of resources out there for what I'm trying to accomplish is slim to none. If they do exist, the people who know tend to be close-lipped or on the opposite side of the spinning sphere we call home.
But here's what you're here for....
✅ PeakeCoin (Staking-Ready, No Rewards Yet)
This contract:
-Tracks staking time per user automatically
-Does not pay out rewards
-Prepares all the data needed to reward users later
-Uses standard ERC20 functions (transfer, mint, burn)
🔧 PeakeCoin.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract PeakeCoin is ERC20, Ownable {
struct StakeData {
uint256 lastUpdated;
uint256 accumulatedTime;
uint256 lastBalance;
}
mapping(address => StakeData) public stakeData;
constructor() ERC20("PeakeCoin", "PEK") {}
function _beforeTokenTransfer(address from, address to, uint256 amount) internal override {
if (from != address(0)) _updateStake(from);
if (to != address(0)) _updateStake(to);
super._beforeTokenTransfer(from, to, amount);
}
function _updateStake(address user) internal {
StakeData storage s = stakeData[user];
uint256 nowTime = block.timestamp;
if (s.lastUpdated > 0) {
uint256 delta = nowTime - s.lastUpdated;
s.accumulatedTime += delta * s.lastBalance;
}
s.lastBalance = balanceOf(user);
s.lastUpdated = nowTime;
}
function getStakeScore(address user) external view returns (uint256) {
StakeData memory s = stakeData[user];
if (s.lastUpdated == 0) return 0;
uint256 delta = block.timestamp - s.lastUpdated;
return s.accumulatedTime + (delta * s.lastBalance);
}
// Optional: mint and burn by owner/bridge
function mint(address to, uint256 amount) external onlyOwner {
_mint(to, amount);
}
function burn(address from, uint256 amount) external onlyOwner {
_burn(from, amount);
}
}
Upvoted! Thank you for supporting witness @jswit.