Python and Network Automation HOW TO - Part 1 Basic Show Commands
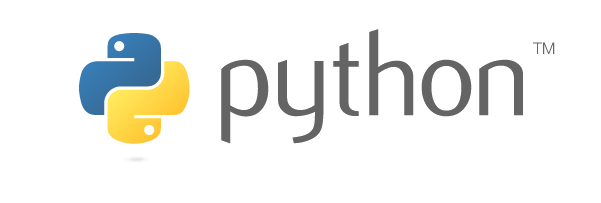
Getting started with automating network tasks using Python
As a network engineer by trade, I'm familiar with networking protocols and their operations as well as managing configurations for devices such as routers, switches, firewalls and others. Lately with the amount of devices that require config changes or even just a basic bootstrapping to get them off the ground for a deployment I've begun using Python3 more and more for this process. With the focus of automation and Software Defined....everything lately I wanted to begin a sort of blogging journey to chronicle the things I'm learning each day and hopefully along the way help out someone else trying to learn.
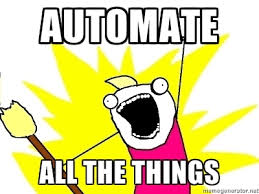
Beginning with Python and Netmiko to perform simple tasks
So to get started I am going to assume a basic level of knowledge of Python data types, dictionaries, lists, and methods as well as a entry level knowledge of Networking such as VLANs, ARP, MAC Addresses and IP Addressing. If this is a topic you would like me to cover in the future as a kind of quick intro to Python or Networking 101 let me know in the comments but for now this is just me working on projects I am covering in my day to day.
We are going to design this simple script to get a command output from a switch in our example and in my next post we can build upon this and run configuration commands to create a new VLAN and tag it to a port. I'm using an Ubuntu 16.04 server to execute the script and an HP Procurve 2530 switch I have to test this out on. Keep in mind the Netmiko library for Python supports a pretty wide list of devices including the following at the current time of this writing:
- a10
- alcatel_sros
- arista_eos
- aruba_os
- avaya_ers
- avaya_vsp
- brocade_fastiron
- brocade_netiron
- brocade_nos
- brocade_vdx
- brocade_vyos
- checkpoint_gaia
- ciena_saos
- cisco_asa
- cisco_ios
- cisco_nxos
- cisco_s300
- cisco_tp
- cisco_wlc
- cisco_xe
- cisco_xr
- dell_force10
- dell_powerconnect
- eltex
- enterasys
- extreme
- extreme_wing
- f5_ltm
- fortinet
- generic_termserver
- hp_comware
- hp_procurve
- huawei
- juniper
- juniper_junos
- linux
- mellanox_ssh
- ovs_linux
- paloalto_panos
- pluribus
- quanta_mesh
- ubiquiti_edge
- vyatta_vyos
- vyos
I am using Python 3.6 in this example but I'm going to try to make this script compatible with Python 2 releases as well in case you are using a system running Python version 2.x. If you want to find out what your system's Python version is using as a default you can run the following command in a terminal window: python --version
Here is the start of the script we will be making, I will go over each line to describe what is going on at each step:
#!/usr/bin/env python
from __future__ import print_function
import netmiko
from getpass import getpass
- #!/usr/bin/env python - This is known as the shebang or bang in Linux scripting, what this is doing is telling the Linux kernel as it begins to read an executable file that this should be run using the Python binary that is defined in the users environment whether that be a version of Python 2 or 3.
- from future import print_function - This is a step to ensure things work for either python 2 or 3, one of the biggest changes between the two versions is how the print function operates. What this does is make the print function in a Python 2 environment operate like the function of Python 3, when Python 3 reads this line it disregards so no harm done.
- import netmiko - This is the library we will be using to get an SSH shell into devices to perform operations
- from getpass import getpass - We don't want passwords being typed in plaintext or stored in plaintext in a file so we import the getpass method from the getpass library so we can prompt for input and not have it show on the terminal as it is being typed.
NOTE You may have to install the netmiko library in your Python environment, you can use something like pip to install this by running in your terminal:
sudo pip install netmiko
Creating the Netmiko SSH Session
So we imported the libraries we need and now it's time to get started working with returning command output from Netmiko. To start we need to build a connection object using Netmiko that will be the interactive ssh shell we use later. We can do this using:
connection = netmiko.ConnectHandler(ip='172.31.1.251',
device_type='hp_procurve',
username='admin',
password=getpass('Enter a password for the switch:\n'))
print(connection)
connection.disconnect()
You can replace the device_type= section with any of the supported types I listed above and of course the username and IP in the example are just filler so put your info in there. The print statement will simply return some info regarding the socket we established and finally we want to disconnect the socket as this is just a simple test to validate functionality. Python's error messages if you encounter any are usually pretty human readable and to the point but common errors would be Host timeout or Authentication failure messages.
If everything goes as expected when you run the script you will get a prompt to enter your password which is what the password=getpass('Enter a password for the switch:\n')
section does. Keep in mind you won't see what you are typing. If things go well after you hit enter you will see something like the following:
Enter a password for the switch:
<netmiko.hp.hp_procurve_ssh.HPProcurveSSH object at 0x0000025C7B41B240>
Getting output from a show command
Now that we validated we can establish a succesful SSH session, let's run a command. We are going to run a simple show version and append the command prompt of the switch to the printed output so we can see the hostname as well. To accomplish this we add to our existing script making it look something like this:
#!/usr/bin/env python
from __future__ import print_function
import netmiko
from getpass import getpass
connection = netmiko.ConnectHandler(ip='172.31.1.251',
device_type='hp_procurve',
username='admin',
password=getpass('Enter a password for the switch:\n'))
print(connection)
result = connection.find_prompt()
result += connection.send_command('show version')
print(result)
connection.disconnect()
Then once we run this we should get output similar to the below, of course it will vary depending on your switch model, etc..

So we've successfully ran a simple show command against our switch, feel free to play around with different commands to get output. In later posts I will get into running commands against multiple devices as well as catching exceptions that may occur while attempting to hit multiple hosts in a script but for now we are on the right foot to get something good started.
Wrapping Up
This is a bit of a departure from my other posts as I stated previously but I plan on doing more tutorials like this as I know Steemit is a great platform to help others develop new skills while honing our own. In the second part I am going to cover executing config commands against a host and we will continue to build upon our script we made today.
Thanks for reading and I hope you found this helpful or interesting. Please feel free to comment with ideas you'd like to see, and don't hesitate to follow or upvote!
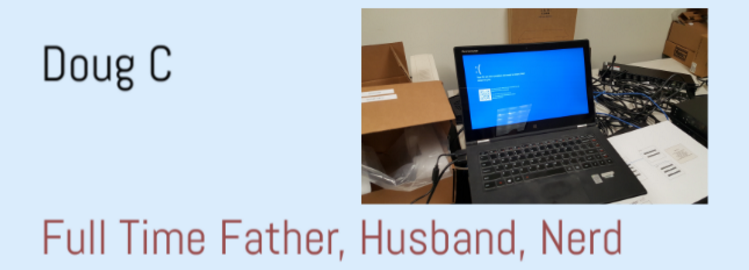
Thanks for this. I am going to play with netmiko this weekend :)
Let me know how it worked out, just posted a second part!
Been playing with it for a while, and indeed the tutorial was helpful. I think now I don't have to search for Python tutors using assignmentcore and try to cope with my college homework on my own.
Nice tutorial, mate! \o/
Thanks, much appreciated!