SLC S22 Week4 || Exception handling in Java
Hello, here we are with week 4 of the Java course by @kouba01, you can check it too here SLC S22 Week4 || Exception handling in Java
and who knows maybe you'll join too.
Let's move to the code part.
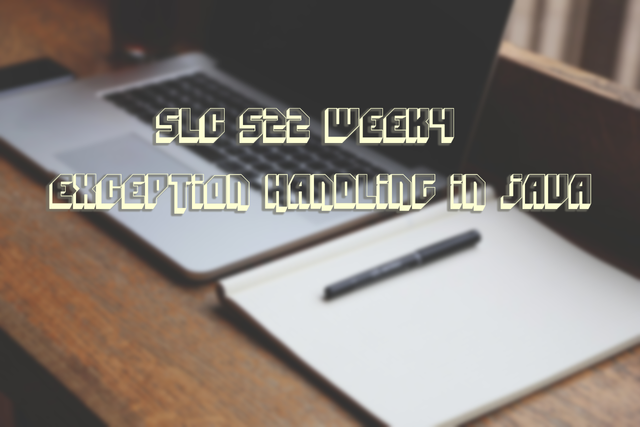
Image taken from Pixabay

You are tasked with managing temperature data collected from multiple sensors in a factory. A sensor may send invalid data (e.g., negative temperatures for a sensor meant to measure above-freezing temperatures). Write a program to process a list of temperature readings and handle exceptions for any invalid values.
Example Explanation
If the sensor provides readings [23, -5, 18, 30], your program should skip the invalid value -5, log an error, and continue processing the other readings. Ensure the program doesn't stop processing all sensors because of one faulty reading.
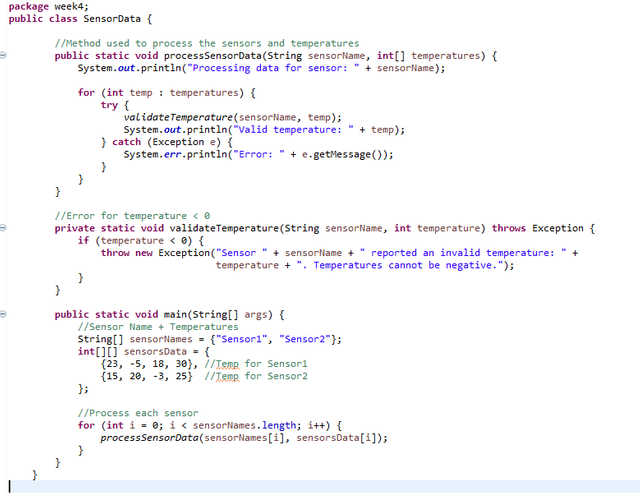
I've created a method processSensorData
that takes the sensor name and an array of temperatures as values, it checks each temperature in a try-catch
block where if the temperature comes out as valid it's printed out further, otherwise it gets the error from validateTemperature
showing which sensor and which temperature are not valid.
To create a grouping with sensor name + temperature I first wanted to use a hash map but I haven't used one for a while so I went with arrays. We have an array for sensor names and an array with temperatures for each sensor, the position of the sensor in sensorNames
has the same position of temperatures in sensorData
.
With a loop we process the sensors and data calling processSensorData
for each sensor and it's temperatures.
For the input presented in the main above we have the following output.
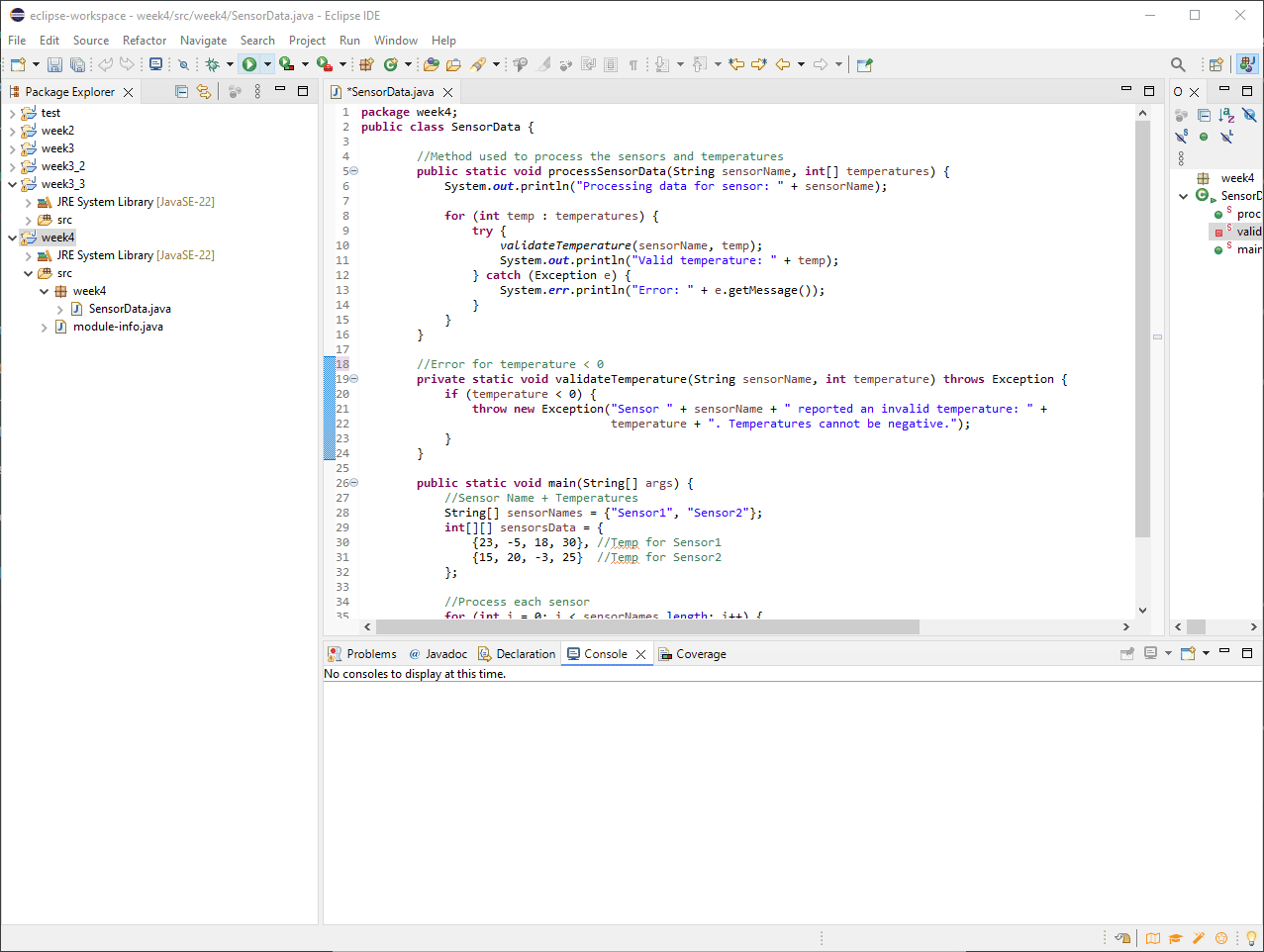


An online registration form accepts participant ages for an event. The age must be between 18 and 60. Write a program that validates the input and throws a custom exception InvalidAgeException for out-of-range ages. Your program should catch the exception and display a message explaining why the input was invalid.
Example Explanation
If a user enters 16, the program should inform them: "Age must be between 18 and 60. You entered: 16." The system should prompt for input again without crashing.
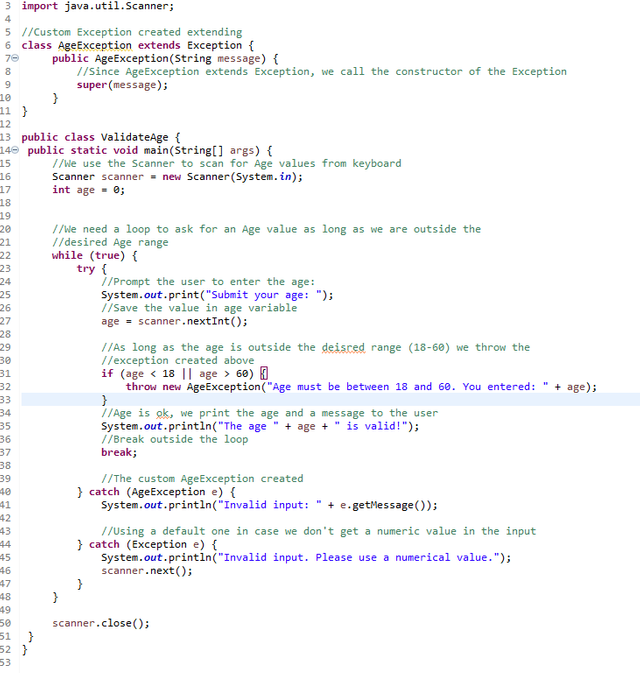
Created a custom Exception class named AgeException
that we are going to use besides the default Exception
one to alert the user when the Age
value is not in the desired range, while the Exception
one will be used to check if we are entering a numeric value.
Using an infinite loop while(true)
we get a value from the keyboard and check it, as long as it is outside the range (18-60) we throw an error and ask the user for a new value. If the right value is provided we break out of the loop using break
.
Let's see a live example:
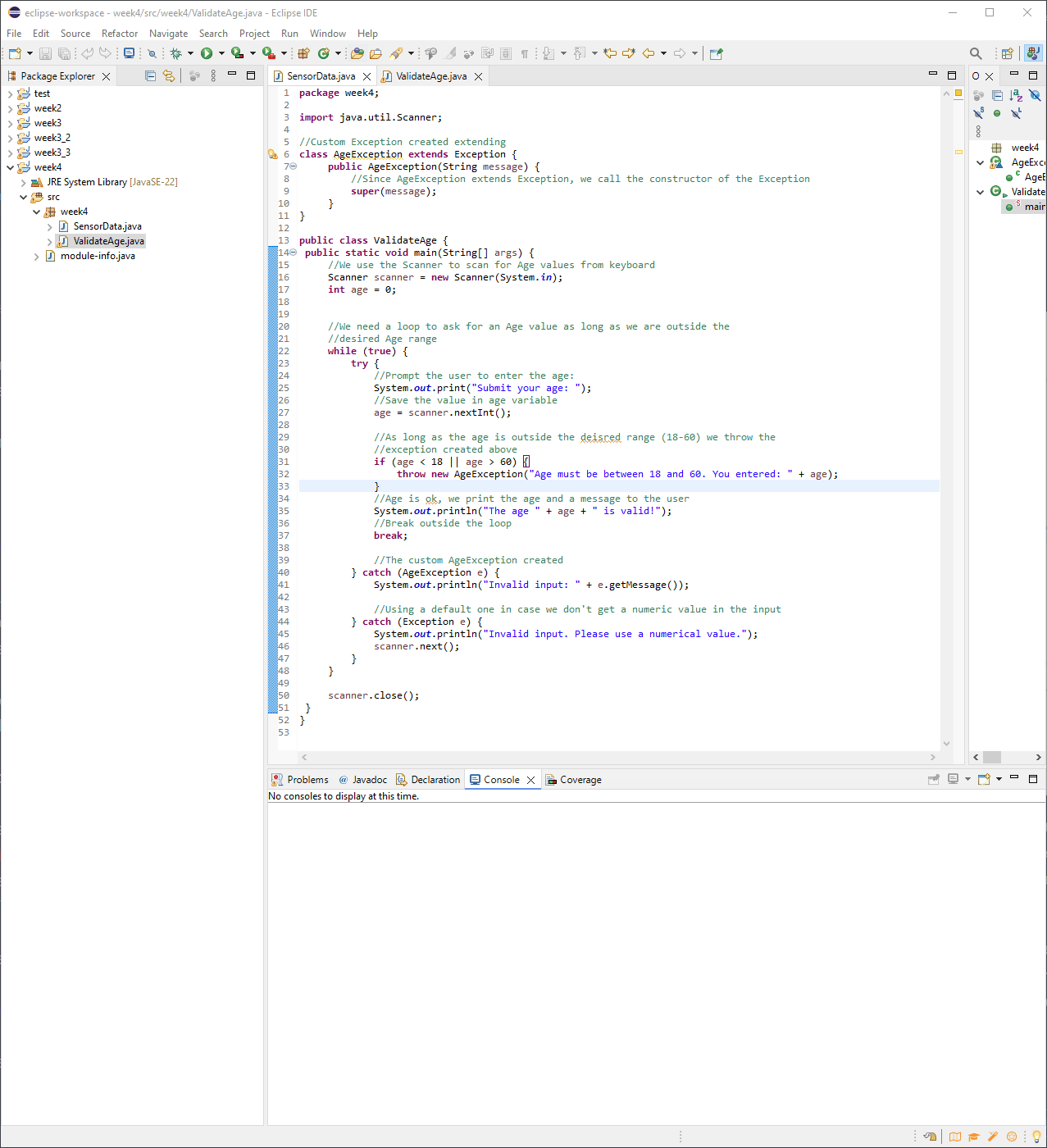


You are developing a simple RPG (Role-Playing Game). Players may encounter various errors such as insufficient health points, invalid moves, or missing inventory items. Design a hierarchy of custom exceptions (e.g., HealthException, MoveException, InventoryException) and write a program to handle them at appropriate levels, depending on the context.
Example Explanation
A player's health drops below zero due to an invalid move. The MoveException should handle the invalid move, and the HealthException should notify that the player is out of health points. Ensure exceptions are handled independently for better game stability.
Since the code in this task exceeds the space of a screenshot I will split it in multiple ones.
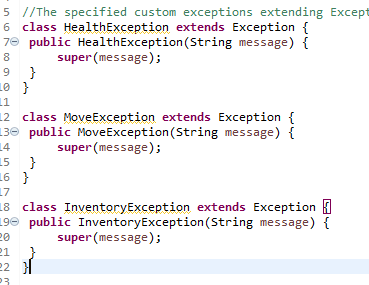
Started by creating the 3 custom Exception, each one extending Exception, HealthException
, MoveException
and InventoryException
.

After that we need some stats for the player that we are going to use through the game to test and throw exceptions. Health
will be the current health of a player, maxHealth
the maximum health capacity while healingPotions
will be the pots available in the inventory.
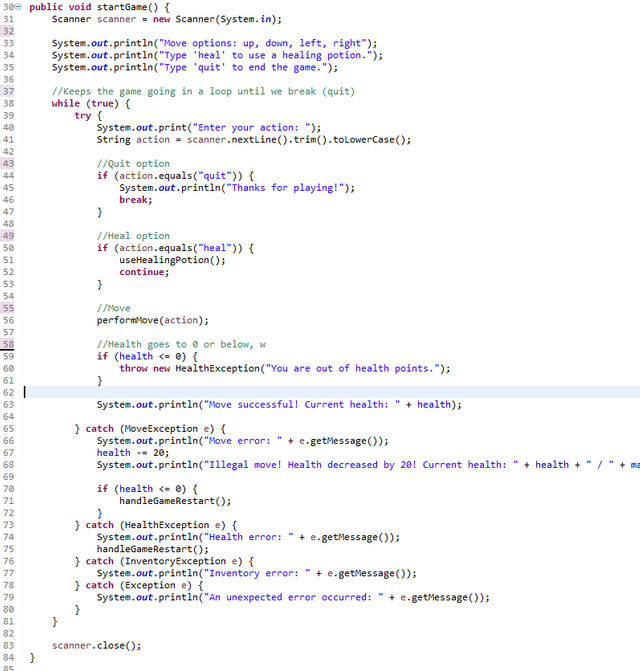
Let's move to the startGame
method, here using a Scanner we are taking inputs from the user and do certain actions based on them. We take the input value in action
and based on it's value we call the corresponding method.
All the actions are taking place in a try-catch
block, each action having it's own exception.
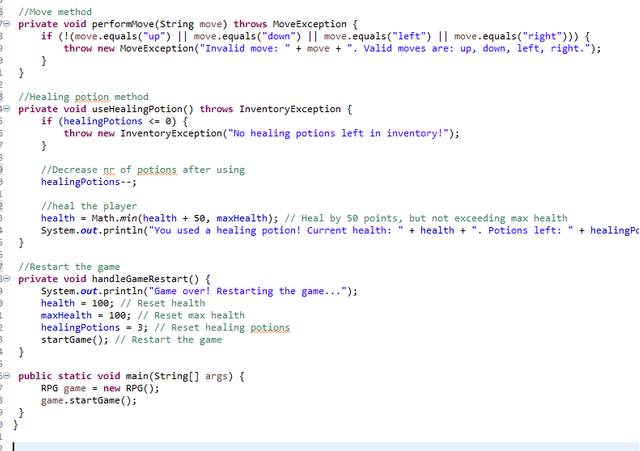
Let's go through the methods:
performMove
allows the user to perform 4 moves (left,right,up,down) anything else would be considered a negative move and throw aMoveException
useHealingPotion
verifies if we have health potions in the inventory, if we have it uses it and decreases it's number, if we don't have it throws theInventoryException
.handleGameRestart
is used to restart the game if we hit 0 health, it resets the player stats and calls thestartGame
method
In the main we create the object and start the game. Let's see a GIF with live example:
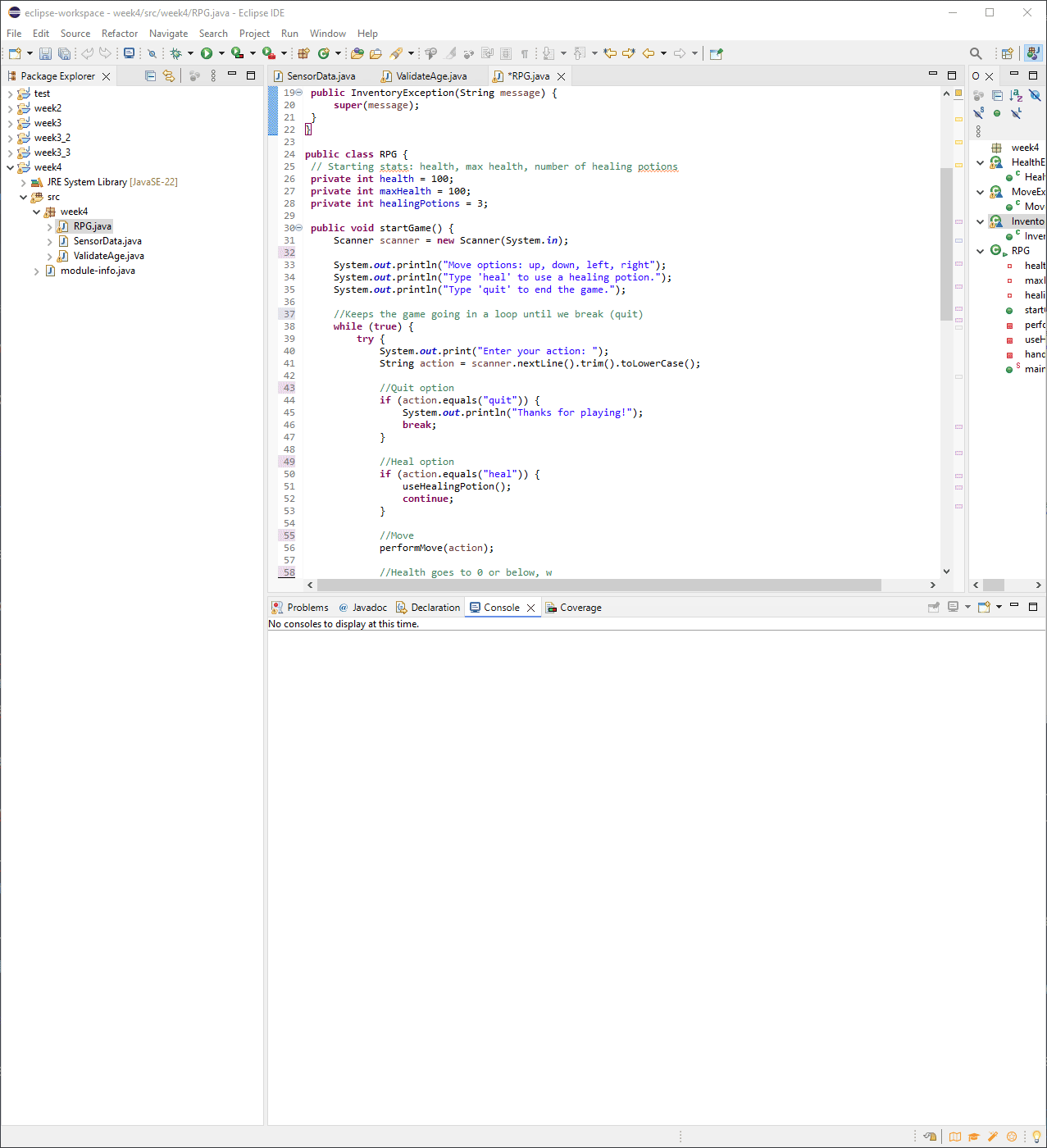
As you can see an illegal move throws an error, health below 0 throws and error and having no more healing pots in the inventory also throws an error.


In a banking system, users can transfer money between accounts. Create a program that validates transactions, handling exceptions such as InsufficientFundsException, InvalidAccountException, and NegativeTransferException. Ensure the program provides detailed feedback to the user and logs errors for failed transactions.
Example Explanation
If a user attempts to transfer -100, the program should catch NegativeTransferException and inform the user: "Transfer amount must be positive. You entered: -100." For an invalid account, display: "Account not found. Please verify the details."
I will also split the code in multiple sections, let's start with the custom exceptions.
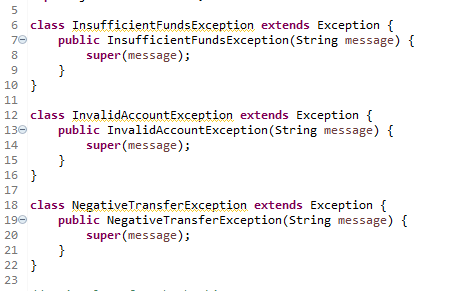
I've created the InsuficientFundsException
, InvalidAccountException
, NegativeTransferException
that extend Exception
.
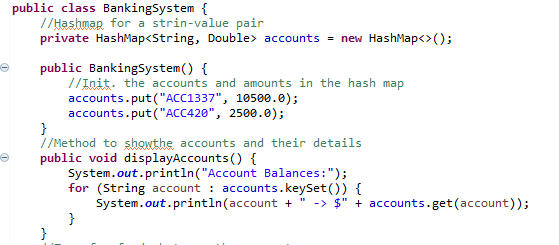
Now for the BankingSystem class, I am going to create a HashMap for a AccountName -> Money couple.
I've added the two accounts ACC1337 and ACC420 to the map with their own money amount, and also created a method to display the accounts and the money each one has.
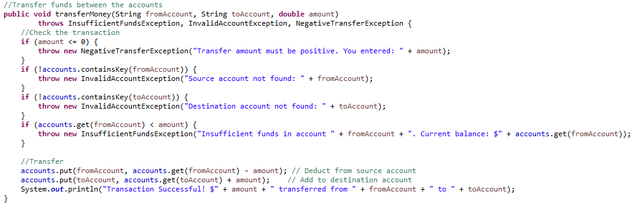
Next on the list is the transferMoney
method that takes arguments the account that sends the money, the receiver and the amount. Here we throw the different errors based on the account name (sender or receiver) and amount.
If the transfer is successful the amount is being deducted and added to the receiver. Transaction being printed at the end, all that we have left now is to create a banking menu.
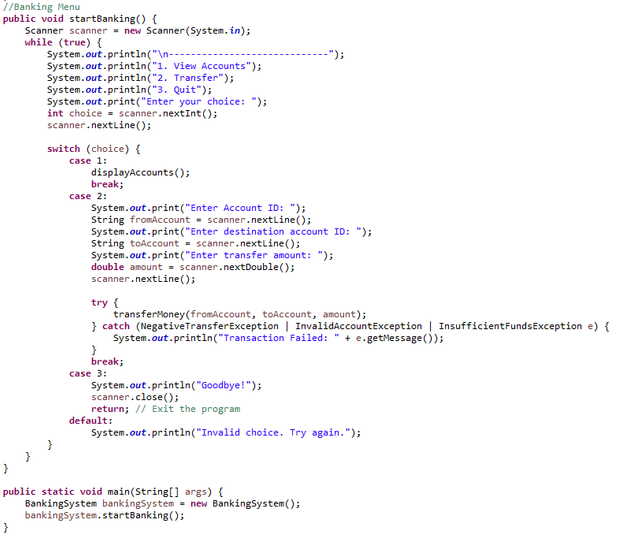
For the menu, I've added everything in a method startBanking
where we present some options to the user and using a switch we move through the options and we can view the details or make a transfer, also there is a quitting option.
Let's check a live example:
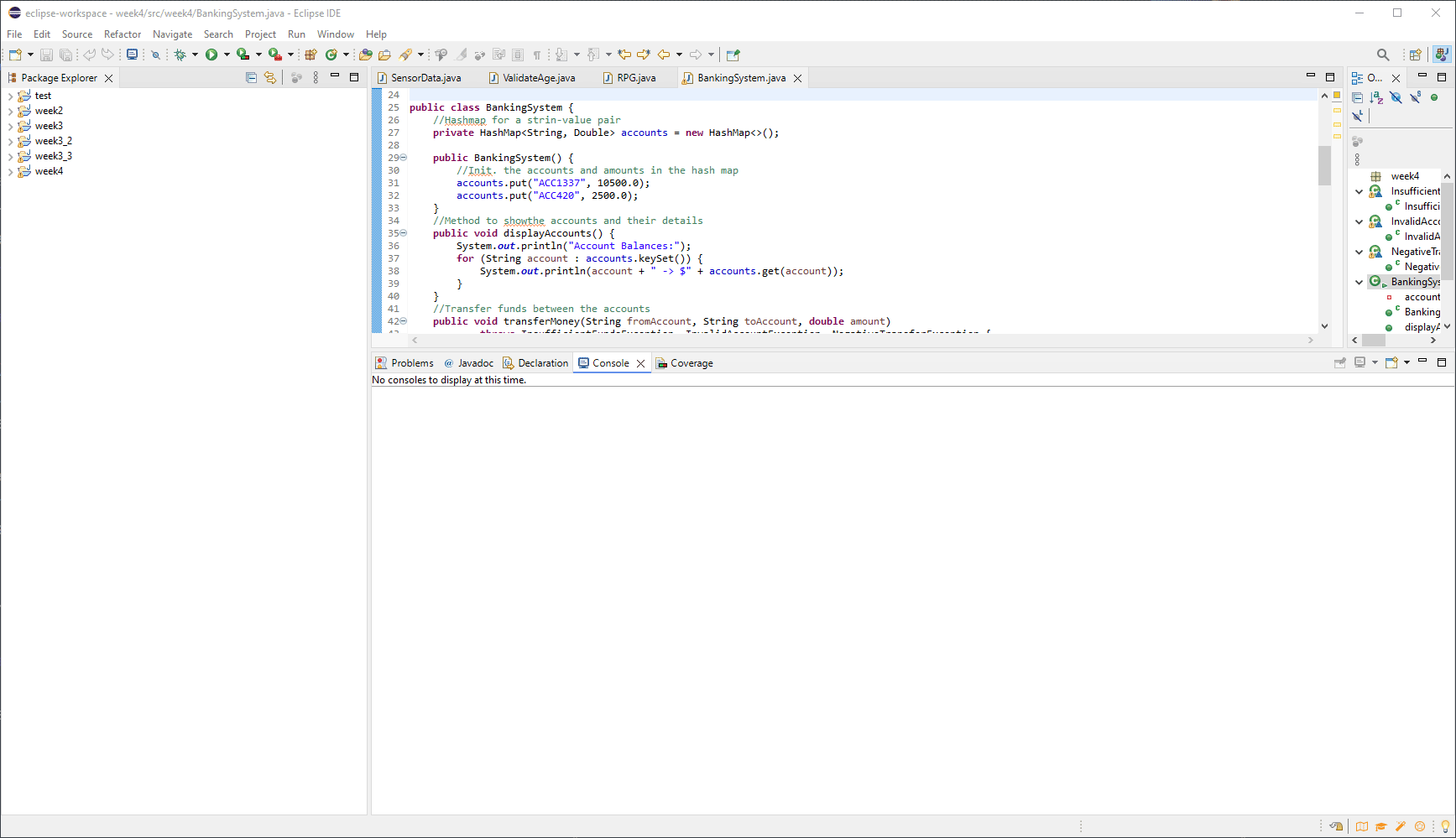
You can see a normal transfer, a transfer where the sender account is wrong, the destination account is wrong, and an invalid amount being sent.
Also forgot to show in the GIF, if the sender account doesn't have the amount that needs to be sent, we get an error.
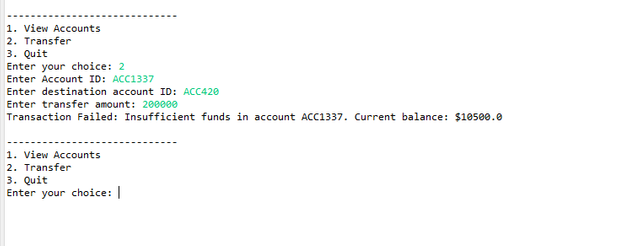


Write a program that opens multiple files, reads their contents, and closes them properly even if an exception occurs during the reading process. Use a finally block or any other mechanism to ensure resource cleanup.
Example Explanation
If a file named data.txt is missing, the program should log: "File not found: data.txt." Regardless of the error, the program should ensure any open files are closed before terminating. For instance, "Closed file: config.txt" should be displayed for successfully handled files.
First we need to create a couple of txt files besides the project in eclipse so once we run the code those can be found, I will use data.txt and validate.txt.
Since we are working with files these time we'll also need some new libraries imported:
- import java.io.BufferedReader;
- import java.io.FileReader;
- import java.io.IOException;
These are used to handle the opening/closing and reading/writing data into files.
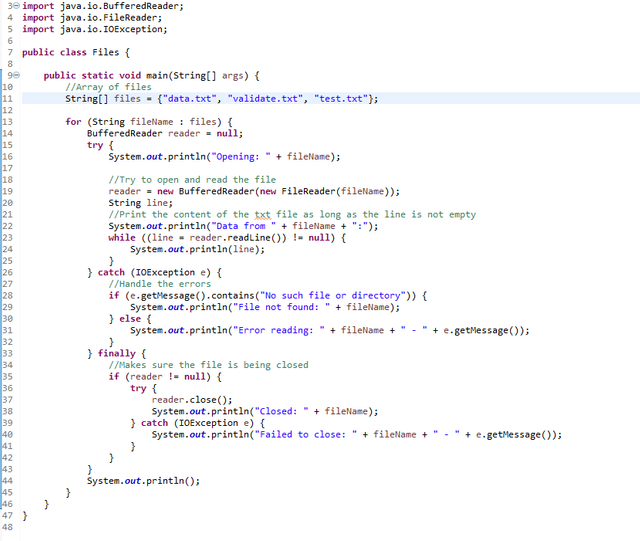
Now let's see how do we handle these files. I created an array of text files to keep track of their names, and for each file in the array we initialize a BufferedReader that will be used to read the data from the file. We read the text line by line as long as the line is not null. If the name doesn't exist we'll throw an error. For the finally
part we check if the BufferedReader is null, if it isn't we attempt to close it, we are trying to close the file, otherwise we throw an error.
And now a live explain in a GIF:
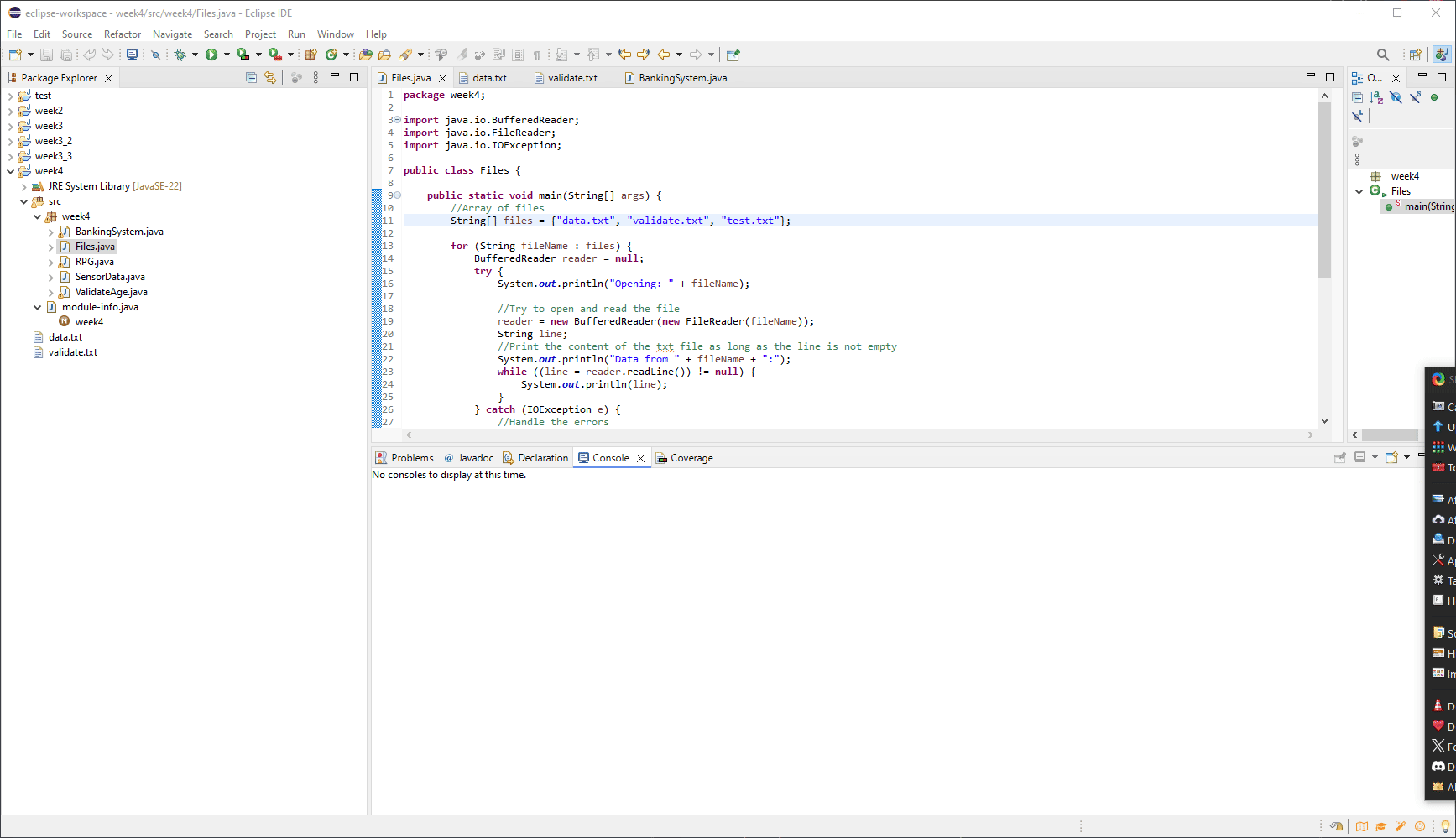


Design a program that processes user activity logs for an e-commerce website. If a user attempts to log in with incorrect credentials more than 3 times consecutively, throw a SuspiciousActivityException. Ensure the program records the activity for further analysis and alerts the administrator.
Example Explanation
If a user attempts logins with passwords abc123, password!, and welcome consecutively without success, the system should raise an alert: "Suspicious activity detected for user: kouba01." Administrators should be able to review these details without halting the service.
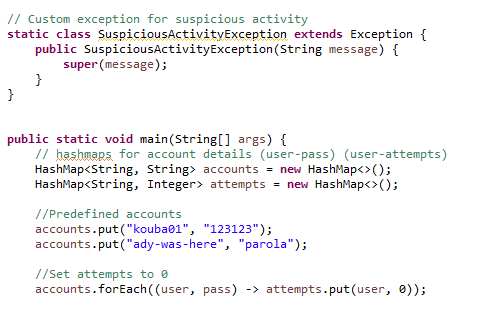
Created the SuspiciousActivityException
that extends Exception, created two hashmaps for username and password and username with the number of attempts for the password. Created two test accounts and set their attempts to 0.
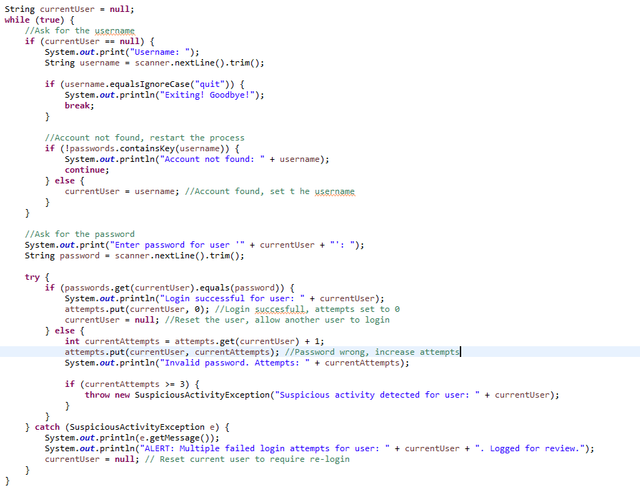
Now we have a currentUser
that is set to null, this variable will be used to try the same password for the same user so we don't have to ask for the username in each attempt.
As long as we are in the while
loop we are checking if currentUser
is null, if null it means we can enter a username to login (or use quit, to quit the menu and get out of loop).
The username exists we ask for the password, if it doesn't exist we let the user know the username is invalid and ask for a new one.
The user has 3 attempts to enter a correct password, each invalid entry throws an invalid password error and if the attempts limit is reached we throw a Suspicious activity error and print an Alert for that user.
A live example:
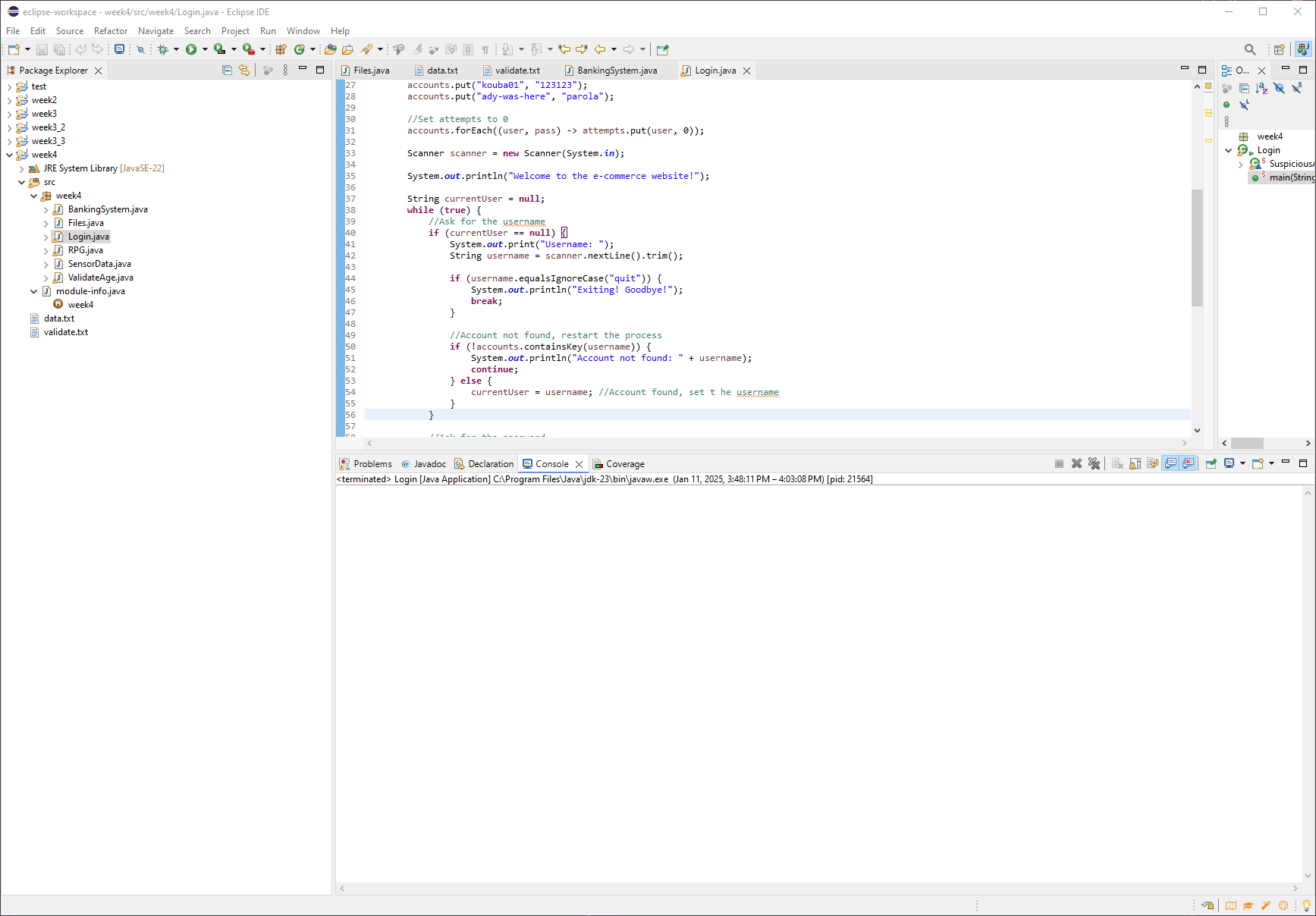
As you can see entering a non existent account tells the user the account was not found, entering a correct one prompts you to enter a password, wrong password tells you the password is wrong and failing it 3 times alerts the admin. A correct username and password results in a successful login.
Also added a quit option that once read in the username will exit the loop.
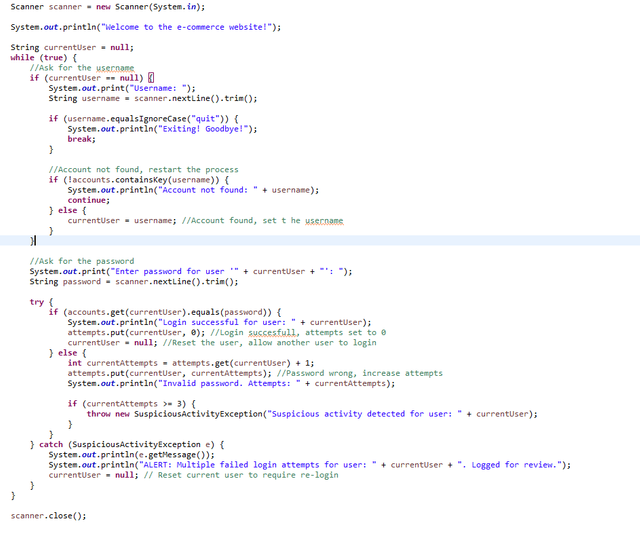
That was it for this Java challenge, can't wait to see what we are facing next week, in the end I'd like to invite @mojociocio @r0ssi and @titans
Thank you for stopping by, wishing you a great day!
Hi @ady-was-here you have shared an amazing post with us implemented all the concepts clearly and presented them well by showing the outputs and live examples.Its impressive you have got full marks Congratulations and Best of Luck.
Thank you :)