SEC-S20W4 Decision Making in Programming: If-Then-Else
🌟 Programming Tasks and Solutions: From Boolean Logic to Algorithm Design 🌟
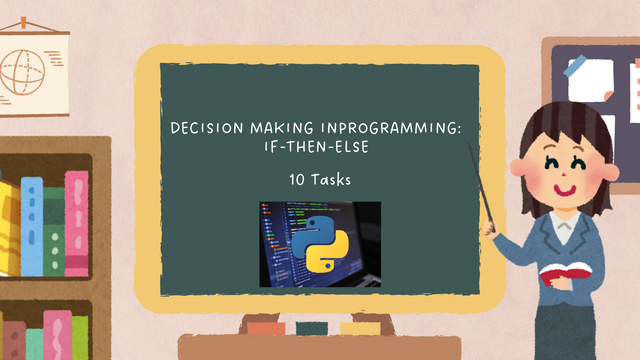
Image Source: canva
In this blog post, we'll explore 10 programming tasks that cover various aspects of coding, from basic concepts to algorithm design. Each task comes with a detailed explanation, solution, and example execution, making it perfect for both beginners and those looking to refresh their skills.
Task 1: Optimal Growth Algorithm
Problem: How can you grow from 1 to any number using only +1 and x2 operations?
Solution (Python Code) :
Explanation of the program:
This algorithm uses a greedy approach to work backwards from the target number. It repeatedly divides by 2 if the number is even and greater than 2, or subtracts 1 otherwise. This process continues until we reach 1. The steps are then reversed to give the sequence of operations from 1 to the target.
Example execution:
Task 2: Boolean Variables
Image Source: canva
Question: What is the purpose of Boolean variables? Why are they called that?
Answer:
Boolean variables are named after George Boole, a mathematician who developed Boolean algebra. They are used to represent two states: true or false (1 or 0). They're essential in programming for conditional logic, flags, and representing binary states.
Task 3: Conditional Operators
Image Source: canva
Question: What is the purpose of a conditional operator? What types exist?
Answer:
Conditional operators are used to make decisions in code based on certain conditions. The main types are:
- If statement (if, else if, else)
- Switch statement (in some languages)
- Ternary operator (condition ? true_result : false_result)
Task 4: Subscription Check Program
Problem: Write a program to check if you've subscribed to 100 users.
Solution (Python Code):
Explanation of the program:
This program takes user input for the number of subscriptions and uses conditional statements to check if the number is equal to, less than, or greater than 100. It then outputs an appropriate message based on the comparison.
Example execution:
Task 5: Steemit Subscribers vs Posts Comparison
Problem: Write a program to check whether you have more subscribers or more posts on steemit.com.
Solution (Python Code):
Explanation of the program:
This program takes user input for the number of subscribers and posts, then compares these two values using conditional statements. It outputs a message indicating whether there are more subscribers, more posts, or an equal number of both.
Example execution:
Task 6: Steemit Triple Comparison
Problem: Write a program to check whether you have more posts, more subscribers, or more Steem Power on steemit.com.
Solution (Python Code):
Explanation of the program:
This program takes user input for the number of subscribers, posts, and Steem Power. It then uses nested conditional statements to compare all three values and determine which one is the highest (or if there's a tie for the highest).
Example execution:
Task 7: Product vs Sum Comparison
Problem: Determine whether the product or sum of two numbers is greater. The user will input the two numbers one by one.
Solution (Python Code):
Explanation of the program:
This program defines a function that prompts the user to input two integers, one at a time. It calculates both the product and the sum of these numbers and compares the two:
- If the product is greater than the sum, it returns a message stating that.
- If the sum is greater than the product, it returns the opposite message.
- If both are equal, it informs the user accordingly.
Example execution:
Task 8: House Number Side Check
Problem: Given two house numbers, determine if they are on the same side of the street (assuming all even numbers are on one side, and all odd numbers are on the other).
Solution (Python Code):
Explanation of the program:
This program defines a function that prompts the user to input two house numbers, one at a time. It uses the modulo operator to check if both house numbers are either even or odd:
- If both numbers yield the same remainder when divided by 2, they are on the same side of the street, and a corresponding message is returned.
- If the remainders differ, it indicates that the houses are on opposite sides of the street.
Example execution:
Task 9: Number Property Check
Problem
Given a number, determine if it satisfies the following conditions:
- It has more than four digits.
- It is divisible by 7.
- It is not divisible by 3.
Solution (Python Code):
Explanation of the Program
- Function Definition: The function
check_number_properties
takes an integernumber
as input. - Condition Check: Inside the function, three conditions are checked using a single boolean expression:
- The number is greater than 9999 (it has more than four digits).
- The number is divisible by 7 (
number % 7 == 0
). - The number is not divisible by 3 (
number % 3 != 0
).
- Conditional Return: The function uses a conditional expression (also known as a ternary operator) to return a string indicating whether the number satisfies all conditions.
- Input and Output: The user is prompted to enter a number, and the function is called with this input to display the result.
Example Execution
Task 10: Two-Digit Number Comparison
Problem
Which digit of a two-digit number is larger—the first or the second?
Solution (Python Code):
Explanation of the Program
- Function Definition: The function
compare_digits
takes an integernumber
as input. - Validation Check: It first checks whether the number is between 10 and 99 (inclusive) to ensure it is a valid two-digit number.
- Digit Extraction:
- The first digit is obtained by performing integer division by 10 (
number // 10
). - The second digit is obtained using the modulo operator (
number % 10
).
- The first digit is obtained by performing integer division by 10 (
- Digit Comparison:
- If the first digit is greater than the second digit
, it returns a message indicating that the first digit is larger.
- If the second digit is greater, it returns a message indicating that the second digit is larger.
- If both digits are equal, it returns a message indicating that they are equal.
- Invalid Input Handling: If the input number is not a valid two-digit number, it returns a message prompting the user to enter a valid number.
Example Execution
Conclusion
These 10 tasks cover a wide range of programming concepts, from basic conditional statements to more complex algorithm design. By working through these problems, you can improve your coding skills and deepen your understanding of various programming techniques. The explanations and example executions provided for each task should help you understand how these programs work in practice.
I want to invite @hudamalik20 , @walictd and @mahadisalim join the contest.
My introduction post on Steemit: link
About the Author
👋 Hi, I’m Kafio!
Software Engineer | Data Science Enthusiast | Trading Buff | Development Geek | Computer Science Lover 💻📊📈
I’m passionate about exploring the intersection of technology and innovation, with a special interest in data science, trading, and software development. Whether it’s diving into the latest in computer science or developing new projects, I’m always excited to learn and share insights. 🚀
I also love to travel
Got questions or just want to connect? Feel free to reach out to me at: [email protected] 🌟
the post is shared on Twitter: Twitter Link.
Hi friend, you have presented well and correctly friend. I think you have no doubt that you are one of the programmers. Good luck my friend. Thanks for the invitation...
Thank you, my friend!
Thank you, @eliany, for your support!
This article is most likely written by an AI, so does it deserve a vote?
Cc. @steemcurator02 @sergeyk
No. I create the content for my posts and use tools to check my grammar. However, there is no tool that can definitively determine whether something was created by AI or not. look is that you text you write the tool saying with ai
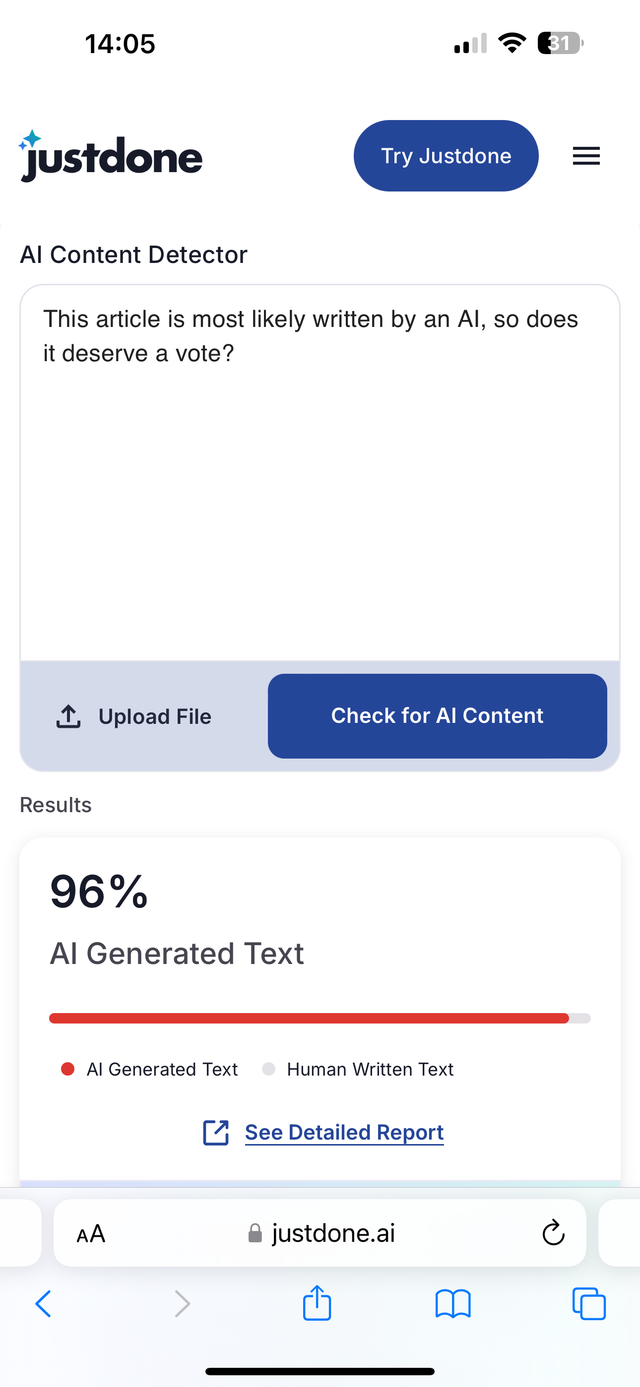