SLC S22 Week4 || Exception handling in Java
🌟 Exception Handling in Java: A Practical Guide 🌟
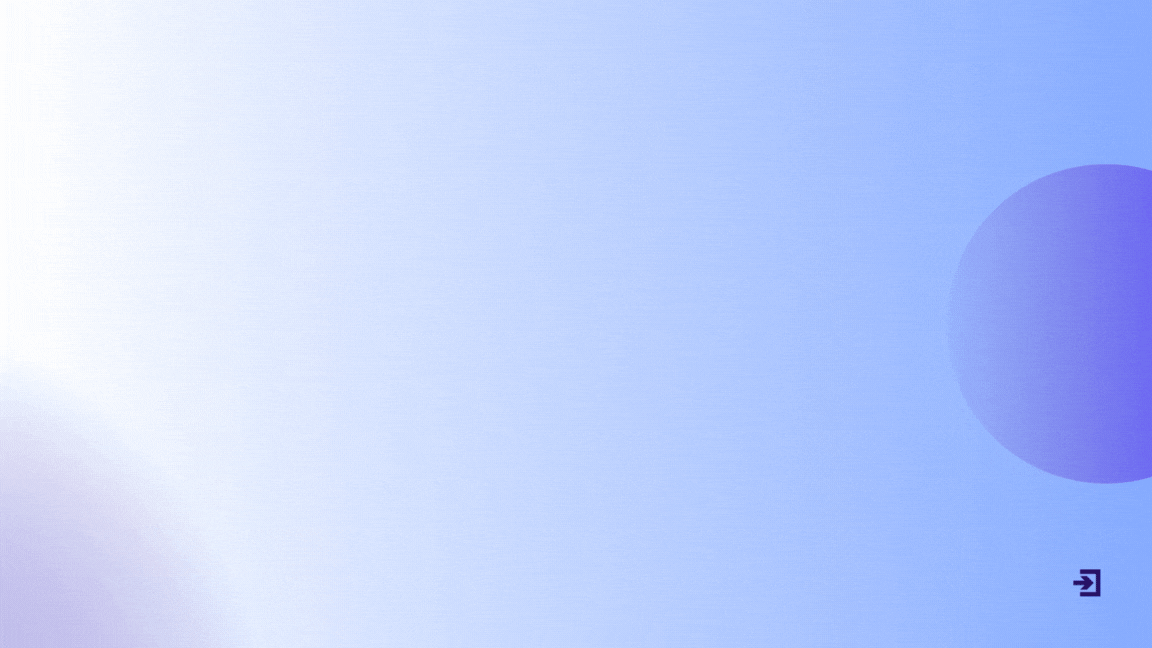
Image Source: canva
Source Code
All code examples are available at: GitHub Repository
Task 1: Detecting Faulty Sensor Readings
Code Overview
this code demonstrates handling invalid temperature readings from multiple sensors the code uses a custom expetison InvalidTemperatureException
to handle negative temperatures, ensuring the system continues processing even when encountering invalid readings.
Key features:
- Custom exception for invalid temperatures from sensors
- ArrayList to store valid temperatures
- Error logging for invalid temperatures
- Continuous processing despite errors
Detailed Code Explanation
// Key components:
1. Custom Exception:
class InvalidTemperatureException extends Exception
- Handles negative temperature values
- Provides detailed error messages
2. Temperature Processing:
processTemperatures(List<Double> readings)
- Takes a list of temperature readings
- Filters out negative values
- Returns only valid temperatures
3. Test Cases:
temperatures1 = [23.0, -5.0, 18.0, 30.0]
temperatures2 = [-23.0, 5.0, -18.0, -30.0]
Testing Results
Task 2: Age Validation System
Code Overview
this program an robust age validation system using custom exceptions it continuously prompts users until valid input is receiv, demonstrat proper exception handling for input validation
Key features:
- Custom InvalidAgeException
- Input validation loop
- Clear error messaging
- Scanner for user input
Detailed Code Explanation
// Core functionality:
1. Age Validation Rules:
- Minimum age: 18
- Maximum age: 60
- Throws InvalidAgeException for invalid values
2. Input Processing:
- Continuous loop until valid input
- Scanner for user input
- Exception handling for non-numeric input
3. Usage Example:
Input: 15 -> Error: Age must be between 18 and 60
Input: 25 -> Success: Age validated
Testing Results
Task 3: RPG Exception Hierarchy
Code Overview
this RPG system implements a hierarchical exception handling structure & multiple custom exception it demonstrat proper exception inheritance and contextual error handling
Key features:
- multiple custom exceptions (healthException, moveException, inventoryException)
- exception hierarchy & gameException as parentes
- interactive game loop
- health monitoring system
Detailed Code Explanation
// System Components:
1. Exception Hierarchy:
GameException (parent)
- HealthException (child)
- MoveException (child)
- InventoryException (child)
2. Game Mechanics:
- Health: 0-100 points
- Actions:
* attack: -50 health
* heal: +30 health
* move: requires health > 20
* use item: inventory check
3. Error Handling:
- Health depletion checks
- Movement validation
- Inventory verification
Testing Results
Task 4: Banking Transaction System
code overview
the banking system implements comprehensive exception handling for various transaction scenarios it ensures safe money transfer & proper validation and error reporting
Key features:
- Multiple banking-specific exceptions
- Balance tracking
- Transaction validation
- Detailed error messages
Detailed Code Explanation
// Banking Features:
1. Exception Types:
BankingException (parent)
-InsufficientFundsException
-NegativeTransferException
2. Transaction Rules:
- Initial balance: 1000.0
- No negative transfers
- Balance must cover transfer amount
3. Error Handling:
- Amount validation
- Balance checking
- Input validation for non-numeric values
Testing Results
Task 5: File Resource Management
Code Overview
this implementaion show proper resource management & multiple files, ensuring proper cleanup using try-&-resources and finally blocks.
Key features:
- multiple file handling
- proper resource cleanup
- error logging
- file processing feedback
Detailed Code Explanation
// Resource Management:
1. File Operations:
- Multiple file reading
- Automatic resource cleanup
- BufferedReader implementation
2. Exception Handling:
- FileNotFoundException
- IOException
- Resource cleanup in finally block
3. Testing Setup:
- Create test files in src/
- Test & existing and non-existing files
Testing Results
Task 6: Security Monitoring System
Code Overview
The security monitoring system tracks login attempts and alerts administrators of suspicious activity. It demonstrates practical application of exception handling in security contexts.
Key features:
- Login attempt tracking
- Admin alert system
- Custom security exception
- User authentication
Detailed Code Explanation
// Security Features:
1. User Management:
- Predefined valid users
- Password verification
- Login attempt tracking
2. Security Measures:
- Maximum 3 login attempts
- Suspicious activity detection
- Admin alert system
3. Implementation:
- HashMap for user storage
- Login attempt counter
- Alert logging system
Testing Results
Conclusion
These implementations demonstrate various aspects of exception handling in Java, from basic input validation to complex resource management and security monitoring. Each example shows how proper exception handling can make applications more robust and user-friendly.
Remember to:
- Always use specific exceptions for different error cases
- Implement proper resource cleanup
- Provide clear error messages
- Log errors appropriately
- Handle exceptions at the appropriate level
I want to invite @livinword, @kingit and @kore-1 join the contest.
My introduction post on Steemit: link
About the Author
👋 Hi, I’m Kafio!
Software Engineer | Data Science Enthusiast | Trading Buff | Development Geek | Computer Science Lover 💻📊📈
I’m passionate about exploring the intersection of technology and innovation, & a special interest in data science, trading, and software development. Whether it’s diving into the latest in computer science or developing new projects, I’m always excited to learn and share insights. 🚀
I also love to travel
Got questions or just want to connect? Feel free to reach out to me at: [email protected] 🌟
the post is shared on Twitter: Twitter Link.