SLC S22 Week4 || Exception handling in Java
Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 22 week 4 by @kouba01 under the umbrella of steemit team. It is about Exception handling in Java. Let us start exploring this week's teaching course.
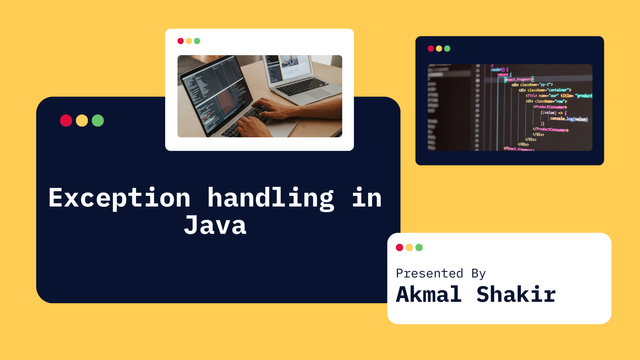
Write a program to process a list of temperature readings and handle exceptions for any invalid values.
Here is the Java program which process a list of temperature readings and handle exceptions for any invalid values.
Explanation of the Code
The program processes temperature readings from the sensors. This program is able to identify invalid values and deal with them in a smooth manner without the program halting.
The program starts with an array of temperature readings temperatureReadings
which simulates data collected from factory sensors. In this case the array contains both valid and invalid readings [13, -5, 5, 0, -7]
. Sensors are expected to record above freezing temperatures so any negative values -5
are considered as invalid.
The program will iterate over every reading in the array using a for
loop. On each reading it checks if it is valid by checking the value:
- A reading which is less than 0 means it is not valid so the program raises a custom exception with the help of
InvalidTemperatureException
. - The custom exception is used to handle specific types of errors pertaining to temperature validation making it more readable and modular.
The code within the try
block attempts to validate each reading:
- For invalid values such as
-5
anInvalidTemperatureException
is thrown with a descriptive error message. - The
catch
block catches the exception and logs the error message for example"Invalid temperature reading: -5"
to the console. This way the program will not crash and will continue processing the next reading.
If a reading passes validation and it is not negative reading it is added to a List<Integer>
called validTemperatures
. This list stores all the valid temperature readings for further use by ensuring that valid data is preserved even when some readings are faulty.
After processing all the readings, the program prints the valid temperatures stored in the validTemperatures
list. This will provide clean output of usable data, ignoring any faulty values.
The program defines a custom exception class as InvalidTemperatureException
by extending Java Exception
class. This custom class allows the program to specify the exact type of error like invalid temperature and makes the code more meaningful and easier to maintain.
In the output of the program it can be seen that there were two values which were negative and these were caught as the invalid values and we got remaining values in the array.
Write a program that validates the input and throws a custom exception InvalidAgeException
for out-of-range ages.
InvalidAgeException
for out-of-range ages. Here is the program that validates the input and throws a custom exception InvalidAgeException
for out-of-range ages.
Explanation of the Code
This program dynamically validates user input for an age-based registration system while ensuring the age is within the range of 18 to 60. If the input is invalid the program throws a custom exception and informs the user of the error and prompts for input again without crashing. This process continues until a valid age is entered.
This program dynamically accepts the user input and for this it initializes a Scanner
to read in user input as follows:
It works by using a while (true)
loop for automatically prompting the user as many times until they type an acceptable answer. This ensures the user is constantly prompted until valid data is entered. The try
block catches any exceptions that may occur during input and validation.
The program checks the input age against the allowed range using an if
condition:
If the age is invalid say below 18 or above 60, then it throws a custom exception InvalidAgeException
with an error message that explicitly describes the reason. This includes the exact invalid age that was input by the user to better communicate to them.
For example in case of invalid age,it catches the exception in the catch block of its respective function.
This block captures the error message to the console using System.err
meaning the program would not crash but allow the user to re-input their age again.
Here is how to handle non-numeric inputs using a general catch
block as well.
For example if the user inputs text rather than a number such as "xyz" this block of code captures the error removes the input from the scanner and allows the loop to move on.
When a valid age that falls within the range of 18–60 is entered by the user the program writes a success message and breaks the loop.
The break
statement ensures the loop stops executing after a valid input and the user is informed that their registration was successful.
A custom exception class InvalidAgeException
is defined to handle age specific errors. This class extends the Exception
class and provides a constructor to initialize the error message.
This makes the program modular and improves the readability of the validation logic.
Overall this program ensures dynamic input validation from numeric to non-numeric with the use of error handling capabilities to prevent failure or crashing as the program calls the user interactively using while (true)
. Practically we can use such systems for the correct input of the age in the registration forms.
Design a hierarchy of custom exceptions (e.g., HealthException, MoveException, InventoryException) and write a program to handle them at appropriate levels, depending on the context.
Here is the Java program which handles the exceptions in the with the help of the custom exceptions:
In this RPG program an error is handled by different exceptions such as an invalid move, not enough health and missing inventory items while playing. This code represents a simple interactive game where players make moves and use items. It handles the exceptional situations without crashing the program.
The program begins by specifying a custom hierarchy of exceptions which is the cornerstone of error handling within specific scenarios. The base class GameException
extends the generic Exception
class and creates specific exceptions depending on the occurrence of various events in the game. The sub-classes of HealthException
, MoveException
, and InventoryException
are more specific types of errors that occur during gameplay.
GameException
: The base class for all exceptions, which accepts custom error messages to be passed and displayed.HealthException
: This exception is thrown when the player's health is not sufficient for a specific action, such as trying to attack when the player has no health left.MoveException
: This exception is thrown when the player makes an invalid move, such as typing an unrecognized action like "fly" instead of "attack" or "retreat."InventoryException
: This exception is used when the player tries to use an item they do not have in their inventory, such as trying to use a gun when it hasn't been acquired.
The RPGGame
Class:
The main class of the game is RPGGame
, which controls the actions and health of the player throughout the game.
The RPGGame
class has two major attributes:
health
: The number of health points the player has. The player begins with 20 health points.hasGun
: A boolean that indicates whether the player has a gun in their inventory, which istrue
at the beginning of this scenario.
The class contains two main methods for the player actions:
makeMove(String move)
This method evaluates whether the player move is valid or not. The program checks in case of move "attack" by verifying whether player has health in his account if player has not the program throws aHealthException
. A player health decreases by 10 for each attack. A game simply acknowledges his retreat action. In case of the invalid moveMoveException
is raised.useItem(String item)
: This method allows the player to use an item. If the player tries to use a gun and does not have it at that time the boolean variablehasGun
isfalse
and anInventoryException
is thrown. If the player tries to use an unrecognized item the program throws anInventoryException
as well. In this case the gun is already availablehasGun
istrue
so the item can be used successfully.
To handle the exceptions in the program in the main there is the implementation of the try-catch
block and finally
block.
- The program catches
MoveException
when an invalid move is encountered and then it prints out the error message. - A
HealthException
is caught whenever a person has less health by displaying an error message. InventoryException
occurs when the required item is not present in the inventory hence it is caught and then the error message is printed.
After each action the program prints "Action completed. You can continue your adventure" by using finally
block. This way the game remains interactive even if an exception occurs. The player can continue playing without the game crashing.
This program demonstrates the use of custom exception handling in a role-playing game. The game checks for errors like invalid moves insufficient health or missing inventory items by throwing and catching specific exceptions. The program ensures the game does not crash and remain interactive even in case of an exception by dealing with each error individually. Using try-catch
blocks meaningful error messages are displayed to the user and the game runs without crashing so that the player can interact with the game at all times.
Create a program that validates transactions, handling exceptions such as InsufficientFundsException, InvalidAccountException, and NegativeTransferException.
Here is the Java program that validates transactions, handles exceptions such as InsufficientFundsException, InvalidAccountException, and NegativeTransferException.
The goal of this banking system program is to handle multiple exceptions that can occur in a financial transaction for example when there are insufficient funds, or invalid account details, or a negative transfer amount. The program will be very interactive and allow the user to simulate a transaction process while ensuring that errors are appropriately handled to avoid system crashes.
The program starts with defining custom exceptions that represent various types of errors that may be encountered during a transaction. Three specific exception classes are defined for handling different error scenarios:
InsufficientFundsException
: This is thrown when a user tries to transfer more money than is actually available in the account.
InvalidAccountException
: When the user input is an invalid account number; that is, an account number that does not exist in the system.NegativeTransferException
: When a user attempts to transfer a negative amount, which is not permissible.
The is the class BankAccount
. The BankAccount
class represents individual bank accounts in the system. Each account has a balance which can be updated through a transaction. The class includes methods for retrieving account details and for transferring money between accounts. The transferFunds()
method is the main logic behind transferring money and is responsible for checking if the account has enough funds and if the transfer amount is valid. Further information of the variables used in this class are given below:
balance
: Represents the current balance of the account.getAccount()
: Retrieves the account based on account number.transferFunds()
: This method checks for insufficient funds and throws anInsufficientFundsException
if the account balance is insufficient. It also checks if the transfer amount is negative throwing aNegativeTransferException
in that case.
The BankingSystem
class models a banking scenario where the users can send money from one account to another. It is comprised of critical methods to retrieve the bank accounts, validate transactions, and handle potential errors that occur in the process of a transaction. The class contains an array of BankAccount
objects representing user accounts. A Scanner
object captures user input of account details and transaction amounts. When a user initiates a transaction, the application checks that the source and destination accounts are valid and carries out all relevant checks before attempting to transfer money.
The functionality of the BankingSystem
class revolves around the getAccount()
method responsible for returning a bank account given its account number. The method iterates through the list of accounts and in case of a match in the account number it returns the corresponding BankAccount
object. In case of no match it throws an InvalidAccountException
to let the user know that the account number is wrong or does not exist. This way only valid accounts can be used for transactions thereby protecting the system from invalid entries.
Next is the processTransaction()
method which serves as the primary entry point to perform a transaction. This method will prompt the user for account numbers and an amount to be transferred. The getAccount()
method will be called to validate both the source and destination accounts. If any account number is invalid an InvalidAccountException
is raised. When valid accounts are retrieved then the method verifies the transfer amount. If the transfer amount is negative, then a NegativeTransferException
is raised if insufficient funds exist in the source account an InsufficientFundsException
is raised. This way only valid positive transfer amounts can be processed with the source account having sufficient balance to complete the transaction.
Upon successful transaction, the method invokes the transferFunds()
method of the BankAccount
class to transfer the given amount from one account to another. After a successful transfer it gives feedback to the user with the new balance of the source account. It catches the specific exception if there are any exceptions like an invalid account or insufficient funds during the transfer process and then prints an error message stating the problem. This ensures that the process of transaction goes smoothly and feedback is given clearly to the user in case of any errors that may occur.
The main
method is the interactive part of the program. There is the functionality to either transfer funds or exit the program, and the user has to give account numbers, amounts to be transferred, and trigger the transaction. In case of invalid input such as an invalid account number or insufficient funds, appropriate exceptions will be thrown and caught in the try-catch
block.
Through this structure the BankingSystem
class ensures the integrity of the banking process. It prevents issues such as negative transfers, invalid accounts, or insufficient funds and provides users with meaningful error messages. This makes the program interactive, reliable and user-friendly by allowing users to perform money transfers without encountering unexpected crashes or issues.
The program simulates a banking transaction system with effective exception handling. It prevents the users from executing invalid actions such as transferring negative amounts, making transactions with inadequate funds and entering invalid account numbers. By throwing and catching custom exceptions such as NegativeTransferException
, InsufficientFundsException
and InvalidAccountException
, the system gives meaningful feedback to the user and prevents the system from crashing during the transaction process. All these functions of the program have been practically shown in the above video.
Write a program that opens multiple files, reads their contents, and closes them properly even if an exception occurs during the reading process.
I will write the program so that opening and reading from multiple files handling common exceptions such as FileNotFoundException
and using a finally
block to ensure that each file is closed correctly. The finally
block is ideal because it runs regardless of whether an exception is thrown or not. This makes it suitable for resource cleanup that files are closed even if an error occurs while reading them.
Here is a Java program that opens multiple files, reads their contents, and closes them properly even if an exception occurs during the reading process.
This program automates Resource Cleanup and reads several files. It is able to handle possible exceptions when the files are not available. It emphasizes proper exception handling for managing file particularly when it comes to resource cleanup on error. This is done using a finally
block to guarantee the proper closing of the files.
The program opens a list of files try to read their contents, and handle cases where files are missing or unreadable. In case of an exception like a FileNotFoundException
an appropriate message will be logged "File not found: < filename >". When the program successfully read files the program will log that the file was read and then closed properly.
Regardless of whether an exception occurs or not the files must be closed after processing. The finally
block is ideal for this purpose. After trying to read each file the finally
block will close the file and print a message saying that the file was closed successfully. This way the program will not leave any file handles open thereby preventing resource leaks.
The program starts with an array of file names (fileNames
). These are the files that the program will try to open and read. Inside the loop a BufferedReader
is opened for each file name to read the file. The program then tries to read every line in the file by using readLine()
. If the file exists and it can be opened for reading. it prints its content to the console.
FileNotFoundException
: This exception is caught if the program could not locate the file for you. In this case, the error message "File not found: < filename >" is printed.IOException
: This exception is caught if there is an issue while reading the file. The error is appropriately printed in this case also.
Regardless of whether an exception has occurred, the finally
block ensures that the file is closed. In case the file was opened without any errors, the program invokes reader.close()
to close the file, and a message is printed indicating that the file has been closed.
- Final Output:
For each file processed, it will output a message either that the file has been read successfully and closed or will log an error message in case the file does not exist or is unreadable.
In the array there are 3 files but one file data.txt
is missing from them. The program is successfully opening, reading and closing the available files and throwing exception for the file data.txt
as it is not found by the program in the directory. The use of the finally
block ensures that no matter what happens resources are cleaned up. In this way we can do file resource management using a program when exceptions can happen.
Design a program that processes user activity logs for an e-commerce website
Here is a Java program that processes user activity logs for an e-commerce website. If a user attempts to log in with incorrect credentials more than 3 times consecutively, throw a SuspiciousActivityException
. The program records the activity for further analysis and alerts the administrator.
This program monitors user login attempts on an e-commerce website and detect suspicious activity. It tracks failed login attempts and raises an alert if a user exceeds three consecutive failed login attempts by signalling potential malicious behaviour. Here is a detailed explanation of how the program works:
The program introduces a custom exception SuspiciousActivityException
, which is thrown when a user makes more than three failed login attempts. This exception allows the program to handle suspicious behaviour in a structured way. The exception class extends the Exception
class, providing a message that can be logged when the exception is thrown.
The main functionality is to check on user attempts to log in. The program starts with a simple login prompt for the user to input a username and password. The correctCredentials
array contains the correct username and password here, "kouba01" and "pass". The program will keep prompting for user login credentials for as long as certain conditions apply.
Each time a user logs in, the checkLogin
method is called to validate the username and password. In case the entered credentials match the correct ones, the login is successful, and the failedAttempts
counter is reset to zero. If the entered credentials are incorrect, the program increments the failedAttempts
counter by one and logs the failed attempt.
In case of a failed login attempt the logActivity
method logs this activity to a log file called user_activity_log.txt
along with a timestamp. This log is saved to a file for later analysis. The log entries contain the timestamp and a description of the activity, thus keeping track of failed login attempts over time.
If the login
method of a user is called three times in a row without any successful login, the program identifies this as suspicious activity. It throws a SuspiciousActivityException
that reflects an error message indicating that there is detected suspicious activity regarding that user. When this occurs, the program logs this suspicious activity and notifies the administrator. In this example, the administrator gets notified through a mock alert message that is printed to the console. In a real-world application, this could be replaced with an email or system notification.
When suspicious activity is detected the program simulates notifying the administrator by printing a message to the console. This action can be extended to send actual alerts, such as email notifications, or log the event to a system that the administrator monitors.
Every failed login attempt is logged with the timestamp, along with the username that attempted to log in. If suspicious activity is detected this is also logged by allowing administrators to review the activity later.
This is the way this program tracks the number of logins prints a failed login to the screen. And then reports suspicious behaviour using a special exception that the application might handle in turn this is confirmed to log the activity. In this program there is a notification for the Administrator in the console about the suspicious activity. The separate file is also taking and recording the activity of the failed attempts for the analysis.
I invite @wilmer1988, @josepha, @wuddi to join this learning challenge.