SLC S21 Week2 || Mastering Arrays with NumPy and Qt5 Design
Hello Steemians
The Dynamic Dev team, as part of Week 2 of the Steemit Learning Challenge Season 21 offers an enriching competition aimed at enabling participants to understand and manipulate one-dimensional and two-dimensional arrays using Python's powerful library, NumPy, all by integrating hands-on exercises to create Python applications capable of elegantly visualizing data using the Qt5 library.
This challenge aims to enable participants to master not only the fundamental and advanced concepts of tables and matrices, but also to strengthen their practical skills by implementing operations on tables and designing interactive and attractive visual interfaces with PyQt5 , while deepening their know-how through concrete and stimulating exercises.
I. Introduction to Arrays
1. Definition and Importance
An array is a structured set of elements that can be accessed and manipulated as a unit to perform various operations in a consistent and efficient manner. Indeed these data structures are particularly useful when storing and processing large amounts of homogeneous data where repetitive and complex operations must be performed efficiently. With their ability to contain multiple elements of the same type under a single entity tables simplify and optimize data management in computer science and programming contexts where performance and code clarity are essential.
2. Why Use NumPy?
The NumPy library is one of the most used in the Python language for numerical and scientific programming, offering a powerful way to manipulate arrays and matrices while providing a set of advanced mathematical functions, unlike the built-in lists of Python, NumPy arrays are designed to execute operations much faster using optimized C code, ensuring more efficient execution.
3. add the NumPy library to the Thonny IDE :
1.Access the Plug-ins Menu:
- Open Thonny and click on the Tools tab in the top menu bar.
- Select the Manage plug-ins... option (see image 1) to access the plug-in management window.
2.Search for the NumPy library:
- In the search bar at the top of the Thonny plug-ins window, type the word numpy and click on the Search on PyPI button to start the search (see image 2).
3.Select NumPy:
- Once the search results are displayed, locate numpy among the listed options and click on it to view more details (see image 3).
4.Install NumPy :
- Click on the Install button at the bottom of the window to begin the NumPy library installation process (see image 4).
5.Installing :
- A pop-up window will open indicating that NumPy is being installed. Wait for the installation to complete (see image 5).
6.Restart Thonny :
- Once the installation is complete, it is recommended to restart Thonny to ensure that the library is properly supported.
II. Introduction to Arrays in Python
Arrays are data structures that allow you to store a set of elements of the same type under the same identifier. In Python, the numpy
library is commonly used to manipulate arrays thanks to its speed and advanced features.
1. One-Dimensional Arrays (Vectors)
1.1. Creating Arrays
To create a one-dimensional array, numpy
offers several methods:
1.2. Accessing Elements
Elements are accessed by their index:
1.3. Manipulating Elements
Arithmetic operations can be applied directly:
2. Two-Dimensional Arrays (Matrices)
When an array consists of simple data types, it is called a one-dimensional array (or vector). When it contains other arrays, it is called a multidimensional array (also a matrix or table). Matrices are thus two-dimensional arrays.
2.1. Matrix Declaration:
All four declarations create matrices initialized with zero values but differ in the type of data stored. The int and float types are used for numeric calculations, while "<U10" and str are used to manipulate strings. The choice between these options depends on the type of data you want to manipulate in the matrix and the application's specific requirements.
2.2.Direct Access:
Direct access means we can get the content of a cell using two values called indices, generally i and j.
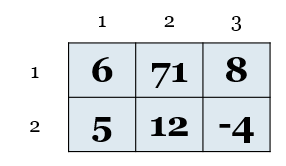
To access a matrix element, its position (row and column) must be specified.
Example: Matrix M [2x3]: M[1, 3] = 8, M[2, 1] = 5.
Another Example: Given the Matrix M (3 x 4) Assign to the variable A, the value of the element found in the 2nd row and the 3rd column?
2.3.Reading/Writing a Matrix:
To read a matrix, all elements need to be traversed to assign a value to each. To display a matrix, each element must be written by traversing the matrix, typically using two nested loops.
M = np.zeros((3, 4), dtype=int)
initializes a matrix of size 3x4 filled with zeros using NumPy, withdtype=int
specifying that the matrix elements are integers.- The first
for
loop iterates through each row (i
), and the nestedfor
loop iterates through each column (j
). - The
input()
function reads integer values entered by the user and stores them in the matrix. - The
print()
statement displays each matrix element with its index.
The +1
in the index display ensures that the output indices start at 1 (to match conventional matrix notation), while NumPy uses 0-based indexing.
2.4. Fill a Matrix :
M = np.zeros((3, 3), dtype=int)
initializes a 3x3 matrix filled with zeros using NumPy, withdtype=int
specifying that the elements are integers.- The first
for
loop fills the matrix by summing the indicesi
andj
, adjusted to start from 1. - The second
for
loop prints the matrix elements with their indices displayed starting from 1 to match the original matrix notation, even though NumPy uses 0-based indexing.
2.5. Calculate the Sum of the Elements in Column C of Matrix M [3,5] (e.g., C = 4):
M = np.zeros((3, 5), dtype=int)
initializes a 3x5 matrix filled with zeros using NumPy, withdtype=int
specifying integer type elements.C = 4
represents the column (using 1-based indexing, corresponding to the 4th column).- The
for
loop iterates over each row of the specified column and adds the element toS
. - The sum is displayed with
print()
to show the result clearly.
2.6. Calculate the Sum of the Elements in Row L of Matrix M [3,5] (e.g., L = 2):
M = np.zeros((3, 5), dtype=int)
initializes a 3x5 matrix filled with zeros using NumPy, withdtype=int
specifying integer type elements.L = 2
represents the row for which the sum is calculated (using 1-based index notation for clarity, corresponding to the 2nd row).- The
for
loop iterates through each column of the specified row and adds each element toS
. - The final sum is displayed using
print()
, showing the total of the specified row.
2.7. Calculate the Sum of All Elements in Matrix M [2,5]:
M = np.zeros((2, 5), dtype=int)
initializes a 2x5 matrix filled with zeros using NumPy, withdtype=int
specifying integer type elements.S = 0
is used to accumulate the sum of all the elements in the matrix.- The nested
for
loops iterate through each element of the matrix to add its value toS
. - The final total sum of the matrix elements is displayed using
print()
.
Homework :
Task1: (2 points)
Create a Python application using PyQt5 that displays Pascal's Triangle based on user input. The program should include the following features:
User Interface (UI):
- Design a GUI window using PyQt5 with an input field where the user can enter an integer
n
(the number of rows for Pascal's Triangle). - Include a table widget to display the triangle and a button that triggers the generation of Pascal's Triangle.
Functional Requirements:
- Write a function to validate the user input, ensuring
n
is a non-negative integer. Display an error message using a pop-up alert if the input is invalid. - Implement a function that generates Pascal's Triangle up to
n
rows:- Set up the first and last elements of each row to
1
. - Calculate the inner elements of the triangle using the sum of the two elements from the previous row.
- Set up the first and last elements of each row to
- Populate the table widget with the values of Pascal's Triangle.
Task2:(2 points)
Write a Python program that reads two integers N1 and N2 (with 2 ≤ N1 ≤ 100 and 2 ≤ N2 ≤ 100) and calculates their product using the "Russian Multiplication" method.
The program should follow these principles: The "Russian Multiplication" method is a technique for multiplying two integers A and B using only division by 2 (integer division), multiplication by 2, and addition. The process involves:
- Dividing the first number (N1) by 2 using integer division and multiplying the second number (N2) by 2.
- Repeating this process until the first number reaches a value of 1.
- Storing the intermediate results in two arrays :
DIVIS
(holding the various values of the first number) andMULT
(holding the corresponding values of the second number). - Summing the values in the
MULT
array that correspond to odd numbers in theDIVIS
array with the second number to get the final product.
Example: For N1 = 11 and N2 = 13, the product is calculated as follows:
The result of the product of 11 and 13 is equal to: 13 + 26 + 104 = 143
Implementation Requirements:
- Create a GUI using PyQt5 where the user can input the two integers N1 and N2.
- Display the calculated product in a designated text field after the user clicks a button.
- Validate the user input to ensure A and B are within the range [2, 100]. If not, display an error message.
- Store the intermediate division and multiplication results in two arrays (
DIVIS
andMULT
). - Implement the calculation of the final result by summing the elements of
MULT
that are aligned with odd numbers inDIVIS
.
Task3: (3 points)
Create a Python application using PyQt5 that allows users to fill, display, and sort a matrix. The application should have the following functionality:
The application should provide a user interface (UI) where the user can input an integer n
to define the size of an n x n
matrix, with n
ranging from 2 to 50. The matrix should be filled with random integer values between 0 and 99. The UI should display the matrix and provide options to sort the matrix either by rows or by columns.
Task4: (3 points)
Create a Python application using PyQt5 that simulates a game where customers can check if their phone number makes them eligible to win a "free cart" or if they need to pay for it. The application should perform the following tasks:
1.Input Validation:
- The user must enter an 8-digit phone number starting with one of the following digits: 2, 3, 4, 5, or 9. If the input is invalid, display an appropriate error message.
2.Array Initialization:
- Implement a function
FillAndDisplay()
to fill an arrayT
of size 8 with random integers between 1 and 99 and display the contents.
3.Digit Sum Calculation:
- Create a function
DigitSum(n)
to calculate the sum of the digits ofn
repeatedly until the result is a single-digit number.
4.Winning Condition:
- Implement a function
Find(x, T)
to check if a specific valuex
exists in the arrayT
. - If the squared digit sum of the phone number exists in
T
, display a message indicating that the customer with that phone number has won a "free cart". Otherwise, display a message stating that they need to pay for the cart.
Note: Please use Thonny and Designer Qt5 to complete all tasks and present the implemented source code,
videos or gifs for completed tasks.
Contest Guidelines
Post can be written in any community or in your own blog.
Post must be #steemexclusive.
Use the following title: SLC S21W2 || Mastering Arrays with NumPy and Qt5 Design
Participants must be verified and active users on the platform.
The images used must be the author's own or free of copyright. (Don't forget to include the source.)
Participants should not use any bot voting services, do not engage in vote buying.
The participation schedule is between Monday, November 04, 2024 at 00:00 UTC to Sunday, - November 10, 2024 at 23:59 UTC.
Community moderators would leave quality ratings of your articles and likely upvotes.
The publication can be in any language.
Plagiarism and use of AI is prohibited.
Use the tags #dynamicdevs-s21w2 , #country (example- #tunisia) #steemexclusive.
Use the #burnsteem25 tag only if you have set the 25% payee to @null.
Post the link to your entry in the comments section of this contest post. (very important).
Invite at least 3 friends to participate in this contest.
Strive to leave valuable feedback on other people's entries.
Share your post on Twitter and drop the link as a comment on your post.
Rewards
SC01/SC02 would be checking on the entire 17 participating Teaching Teams and Challengers and upvoting outstanding content. Upvote is not guaranteed for all articles. Kindly take note.
At the end of the week, we would nominate the top 4 users who had performed well in the contest and would be eligible for votes from SC01/SC02.
Important Notice: The selection of the four would be based solely on the quality of their post. Number of comments is no longer a factor to be considered.
Best Regards,
Dynamic Devs Team
https://steemit.com/dynamicdevs-s21w2/@akmalshakir/slc-s21-week2-or-or-mastering-arrays-with-numpy-and-qt5-design
Here is my entry: https://steemit.com/dynamicdevs-s21w2/@mohammadfaisal/slc-s21w2-or-or-mastering-arrays-with-numpy-and-qt5-design
Greeting sir
Is there any other solution to solve this task? My Laptop does not support Thonny with PyQt5, so I can't complete my previous task.
My entry.
https://steemit.com/dynamicdevs-s21w2/@josepha/slc-s21w2-or-or-mastering-arrays-with-numpy-and-qt5-design