SLC S21W2 || Mastering Arrays with NumPy and Qt5 Design
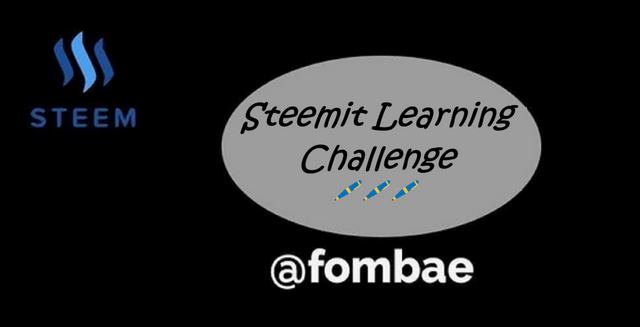
Greetings Steemit friends
Create a Python application using PyQt5 that displays Pascal's Triangle based on user input.
To achieve this, I will start by designing a user interface where the user will be able to input a number for Pascal's triangle. We start with drag and drop QLineEdit, where the user can input the number. Drag and drop QPushButton, one with the text Fill, another with test rest, and the last one with the text Quit. The last and most important widget is the QtableWidget, which displays the triangle.
Next, we implement the function to generate Pascal's triangle up to the n rows and return the list of lists. The next function will populate the table with values generated from Pascal's triangle.
Now, make sure that the input is not a negative value. We used an if statement to check if the number inputted is negative. If it is, an error message will pop up.
Just for fun, I maintain the reset and quit functions that we encountered during the last class. The reset button clears the table and input, while the quit button closes the program.
Write a Python program that reads two integers N1 and N2 (with 2 ≤ N1 ≤ 100 and 2 ≤ N2 ≤ 100) and calculates their product using the "Russian Multiplication" method.
Let's start by designing the user interfaces in which the user will be able to input the two integers N1 and N2. We start with dragging and dropping three QLineEdit, where the user can input the number N1, and N2 and the last one will display the product. Drag and drop three QPushButton, one with the text display, another with text reset, and the last one with the text Quit.
Now, let's move on to the Thonny application and create a new file. I will start with the function to handle Russian multiplication. I set the variables to store the values of N1 and N2 as DIVIS and MULT. The last variable product will be used to hold the final result.
As required by the task, I used a loop to make sure the N1 was greater than 1 and repeated the process to get the value less than 1. The next check now is if the final result of n1 is odd, then add N2 to the product.
The next function is the Display function. Here we will read the values inputted n1 and n2 by the user. Check if the input values are in the range of 2 to 100 as required in the task. Now we call the russian multiplication function and pass the parameters to have the product.
Just for fun, I maintain the reset and quit function which we came across during the last class. The reset button is used to clear the input, while the quit button is used to close the program.
Create a Python application using PyQt5 that allows users to fill, display, and sort a matrix.
Just like the previous task, I start by designing the user interfaces in which the user will be able to input integers N. Drag and drop four QPushButton, one with the text Fill, one for the text sort, another with text reset, and the last one with text Quit. The last and most important widgets are the QtableWidget to display the original matrix and the other to display the sorted matrix.
We move to the Thonny application, the first function is to generate the random integer with the range 0 and 99. The next function puts together the matric dimensions and creation of the cell items for the matrix elements.
Next are the functions the radio button uses to sort by row and sort by column.
The next function Filltable, populates the first table with the random matrix generated from the integer input by the user.
The Sorttable function checks which radio button is selected and populates the second table sorting by row or column.
Just for fun, I maintain the reset and quit function which we came across during the last class. The reset button is used to clear the input, while the quit button is used to close the program.
Create a Python application using PyQt5 that simulates a game where customers can check if their phone number makes them eligible to win a "free cart" or if they need to pay for it.
I started with designing the user interfaces, dragging and dropping QlineEdit for phone numbers to be input. Next is the QpushButton with the text Play, the others with the text Reset, and the last one with the text Quit. I added a QtextEdit to display the messages to the user.
Now let's move on to the Thonny application to complete the operations. We start with the first function, which is to validate the phone number inputted by the user. Check if we have up to eight digits, and start with digit 23459.
Next, to generate the random arrays of numbers. I create the function to generate the random integer in 1 to 99 within the ranges of 8 digits. Next, I calculate the digit sum for the numbers until they become a single digit.
Most important is the File and Display function which we will make use of the previous functions. The variable phone holds the number input, which is then checked to be a valid phone number. Now we generate an array of 8 digits made up of random numbers within 1 and 99. Get the single digit and the square of the digit sum.
Let's check if the phone number is a valid winner of a free cart or not. So we check the digit sum value in the array of 8 elements made of a single digit. If a case is found, the message pops up else the phone number needs to be paid for for the free cart.
Ending, I maintained the reset(delete) and quit functions that we came across during the last class. The delete button is used to clear the input, while the quit button is used to close the program.
This has been a super exciting week and a more challenging task. I am achieving my objective for this course. I will encourage Steemians who are in the It domain to develop some interest in this course.
Cheers
Thanks for dropping by
@fombae
Upvoted! Thank you for supporting witness @jswit.