SEC S20W6 || Date & Time Types And String Predefined Methods in PHP
Hello steemians and welcome to the 6th and final week of Season 20 of the Steemit Engagement Challenge!
This week, we challenge you to work on two fundamental aspects of PHP: date and time management and string manipulation. Showcase your skills by applying these essential functions to create dynamic and efficient solutions.
Good luck to everyone!
Functions Related to Date &Time Types
In order to fully grasp date manipulation in PHP, it is necessary to understand what a Unix timestamp is. Also called Unix time or epoch time, the Unix timestamp represents the number of seconds that have elapsed since January 1st, 1970 at midnight GMT. This date marks the beginning of the Unix era. Indeed, Unix systems record the current date and time as a 32-bit integer.
Knowing the timestamp of a given moment allows you to work with it to obtain all sorts of information: verify the validity of a date, rank members by registration date, etc.
In some dynamic websites, one might need to:
- Assign the system date and/or time to a variable or a field,
- Add a period to a date field,
- Change the display format of a date,
- Process fields containing dates,
- Etc.
1. The checkdate
function
It returns TRUE
if the date represented by the day j
, month m
, and year a
is valid; otherwise, it returns FALSE
.
Note: The argument order is not in the French format. The date is considered valid if:
- The year is between 1 and 32767 inclusive,
- The month is between 1 and 12 inclusive,
- The day is within the date range of the month.
Leap years are considered.
Syntax: int checkdate (int m, int j, int a)
Example:
$j = 30; $m = 11; $a = 2012;
if (checkdate($m, $j, $a) == true)
$d = $j . "/" . $m . "/" . $a;
else
$d = "Invalid data";
echo($d);
2. The date
function
It returns a date as a string, in the format given by the format
parameter. The date used is provided by the timestamp
parameter or the current date and time if no timestamp is provided.
Syntax: string date (string format, [int timestamp])
Example:
$d = date("Y-m-d H:i:s");
echo("Today is: " . $d);
3. The getdate
function
It returns an associative array containing the date and time information of the timestamp
when provided, or the current local date/time if no timestamp is given.
Note: The array contains the following elements:
seconds
,minutes
,hours
,mday
,wday
,mon
,year
,yday
,Weekday
,month
Syntax: array getdate ([int timestamp])
Example:
$a = getdate();
$b = date("d-m-Y H:i:s");
echo("Today is: " . $b . "<br>");
echo("Seconds: " . $a["seconds"] . "<br>");
echo("Minutes: " . $a["minutes"] . "<br>");
echo("Hours: " . $a["hours"] . "<br>");
echo("Day of the month: " . $a["mday"] . "<br>");
echo("Day of the week: " . $a["wday"] . "<br>");
echo("Month: " . $a["mon"] . "<br>");
echo("Year: " . $a["year"] . "<br>");
echo("Day of the year: " . $a["yday"] . "<br>");
echo("Weekday name: " . $a["weekday"] . "<br>");
echo("Month name: " . $a["month"] . "<br>");
4. The time
function
It returns the timestamp, which is a long integer representing the current time, measured in seconds.
Syntax: int time (void)
Example:
$a = time();
$b = date("d/m/Y H:i:s", $a);
echo($a . " = " . $b);
5. The mktime
function
It returns a timestamp corresponding to the given arguments. Arguments can be omitted from left to right, and missing arguments are replaced with the current time and date values.
Syntax: int mktime (int hour, int min, int sec, int month, int day, int year, int is_dst)
Note: is_dst
can be set to 1 if daylight saving time applies, 0 if it does not, and -1 (the default) if unknown.
Example:
$h = 12; $mn = 1500; $s = 560; $j = 300; $m = 21; $a = 2010;
$ns = mktime($h, $mn, $s, $m, $j, $a);
echo("The number of seconds is: " . $ns . "<br>");
$d = date("d-m-Y H:i:s", $ns);
echo("This number of seconds corresponds to the date: " . $d);
6. The strtotime
function
It attempts to read a date in English format and convert it into a timestamp, relative to the now
timestamp, or the current date if omitted.
Syntax: int strtotime (string time, int now)
Example:
$a = strtotime("now");
$b = strtotime("10 September 2000");
$c = strtotime("+1 day");
$d = strtotime("+1 week");
$e = strtotime("+1 week 2 days 4 hours 2 seconds");
echo(date("Y-d-m H:i:s", $a) . " = " . $a . " seconds<br>");
echo(date("Y-d-m H:i:s", $b) . " = " . $b . " seconds<br>");
echo(date("Y-d-m H:i:s", $c) . " = " . $c . " seconds<br>");
echo(date("Y-d-m H:i:s", $d) . " = " . $d . " seconds<br>");
echo(date("Y-d-m H:i:s", $e) . " = " . $e . " seconds<br>");
7. Definition of a timestamp:
A timestamp is a long integer that contains the number of seconds since the start of the UNIX epoch (January 1, 1970 00:00:00 GMT).
Note: The list of functions is not limited to those mentioned; others include gettimeofday
, gmdate
, gmmktime
, gmstrftime
, localtime
, microtime
, and strftime
.
String Predefined Methods
In this second part we explore the key functions of PHP for manipulating strings, allowing you to optimize your code, minimize errors and improve the quality of your PHP developments.
1. The strlen
function
It returns the length of a string.
Syntax: int strlen (string str)
2. The strpos
function
It searches for the first occurrence of a character in a string and returns the numeric position of the first occurrence of ch1
in the string ch
starting from a given position.
Syntax: int strpos (string ch, string ch1, int position)
3. The trim
function
It removes spaces from the beginning and end of a string.
Syntax: string trim (string str, " ")
4. The strrev
function
It reverses the order of characters in a string.
Syntax: string strrev (string ch)
5. The strtolower
function
It converts all characters in a string to lowercase.
Syntax: string strtolower (string ch)
6. The strtoupper
function
It converts all characters in a string to uppercase.
Syntax: string strtoupper (string ch)
7. The str_replace
function
It replaces all occurrences of a string with another string.
Syntax: string str_replace (string search, string replace, string subject)
8. The substr
function
It extracts a portion of a given string.
Syntax: string substr (string ch, int pos, int nbre)
9. Concatenation
String concatenation is done with the operator .
(dot).
Displaying rows in a table in PHP
Explanation:
- This script connects to a MySQL database (with the database name
Database_Name
). - It selects three columns (
column1
,column2
,column3
) from the specified table (TABLE_NAME
). - The data is fetched row by row using a
while
loop, and each record is displayed in a table with three columns. mysqli_fetch_array()
is used to retrieve the records and display the values fromcolumn1
,column2
, andcolumn3
.
Also, note the remark at the end:
- You must close the PHP code whenever switching to write HTML code.
Homework :
Task1: (1points)
Here’s a multiple-choice quiz (QCM) focused on PHP date and time functions, indicate the correct answer, and explain your choice.
1. What does the checkdate
function do in PHP?
- A. It formats a date into a specific string.
- B. It checks if a date is valid based on the day, month, and year.
- C. It returns the current timestamp.
- D. It adds a period to a date field.
2. What does the date
function return if no timestamp is provided?
- A. The system's current date and time as a formatted string.
- B. The time elapsed since 1970 in seconds.
- C. The timestamp for midnight of the current day.
- D. A boolean value indicating whether the current date is valid.
3. Which of the following is true about the mktime
function in PHP?
- A. It returns the current system timestamp.
- B. It generates a timestamp based on provided hour, minute, second, day, month, and year values.
- C. It checks the validity of a date.
- D. It converts a timestamp into a human-readable string.
4. What is the purpose of the strtotime
function in PHP?
- A. It formats a date according to a specific format.
- B. It converts an English date string into a Unix timestamp.
- C. It compares two date strings.
- D. It checks if a date is in the future.
Task2: (3points)
By applying String Functions, complete each code in the table below to achieve the requested result:
Project: (6 points)
A company provides its clients with a leisure equipment rental service. For this, a simplified version of a website is proposed that allows:
- The rental of equipment,
- The recording of the return of rented equipment,
- The editing of daily rental statistics.
Task Required:
A- Creation of the Database
- Create a database named "EquipementLeisure".
- Create all its tables.
- Establish the relationships between the different tables by referring to the following textual representation:
Client (Ncin, Last Name, First Name, Phone Number)
Equipment (Ref, Label, Hourly Price, Available)
Rental (NcinClient#, RefEquipment#, DateRent, DateReturn)
The tables below indicate the description of the tables.
Client Table
Field | Type |
---|---|
Ncin | String of 8 characters |
Name | String of 20 characters |
First Name | String of 20 characters |
Phone Number | String of 8 characters |
Equipment Table
Field | Type |
---|---|
Ref | String of 5 characters |
Label | String of 30 characters |
Hourly Price | Integer |
Available | Character ('O' for Yes / 'N' for No) |
Table Location
Field | Type |
---|---|
NcinClient | String of 8 characters |
RefEquipment | String of 5 characters |
DateLoc | Date and time |
DateRet | Date and time |
NB:
The field "Available" accepts the value 'O' (Yes) when the equipment is available, or 'N' (No) otherwise.
The field "DateRet" indicates the expected return date of rented equipment.
- Insert four records of your choice into the Client table.
- Insert the following rows into the Equipment table:
Ref | Label | Hourly Price | Available |
---|---|---|---|
Jsk01 | Jet-Ski | 25 | 'O' |
Pch76 | Parachute | 15 | 'N' |
Ped01 | Individual Pedal Boat | 10 | 'N' |
Ped02 | Double Pedal Boat | 18 | 'O' |
B- Creation of Web Documents
1)The index page of the website
a) Create the "index.html" page by respecting the following layout:
Details:
- Zone1: An element representing the website header, containing the text "Leisure Equipment Rental" as a level 1 heading.
- Zone2: An element representing the navigation panel, containing the following hyperlinks:
- Rental of equipment (this will link to the page "Location.html").
- Return of equipment (this will link to the page "Return.html").
- Statistics (this will link to the page "Statistics.html").
- Zone3: An element representing the display area for the different web pages, containing by default the page "Location.html."
- Zone4: An element representing the website footer, containing the following text: "Contact us at 33 100 100."
Here is the translation of the content from the image:
2)Create the following form on the "Location.html" page.
Clicking the "Validate" button triggers:
A PHP script developed in the "Location.php" file that performs the following:
- Displays the message "Ncin not found" if the entered ID number does not exist in the Client table.
- Displays the message "Equipment not available" if the selected equipment is unavailable.
- Otherwise, it adds the necessary data to the Location table, updates the "Available" field in the Equipment table, and finally displays a confirmation message.
NB:
- The value of the rental date is equal to the system date.
- The return date is calculated by adding the selected duration to the rental date.
3)Create the following form on the "Return.html" page.
Clicking the "Validate" button triggers:
A file "Retour.php" that allows:
- Updating the "Available" field in the Equipment table and displaying a confirmation message of the operation.
- Alternatively, displaying an error message indicating any issue encountered.
4)Create the page "Statistics.php" that displays the details of all rentals made on the current date, as follows:
- Fields to display:
"Label," "Date of Rental (DateLoc)," "Return Date (DateRet)," "Hourly Price (PrixHeure)," "Amount"
NB: The amount is calculated as the duration of the rental multiplied by the hourly price.
Example of execution
The rental page
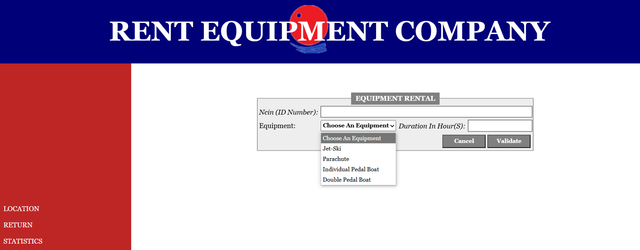
The return page
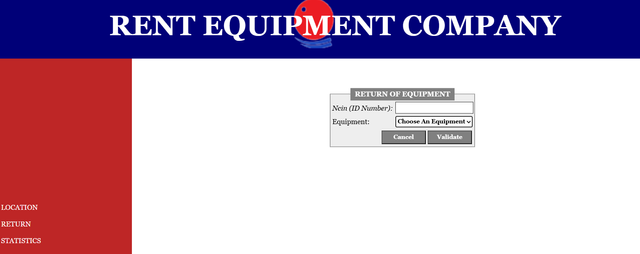
The statistic page
Contest Guidelines
Post can be written in any community or in your own blog.
Post must be #steemexclusive.
Use the following title: SEC S20W6 || Date & Time Types And String Predefined Methods in PHP
Participants must be verified and active users on the platform.
Post must be more than 350 words. (350 to 500 words)
The images used must be the author's own or free of copyright. (Don't forget to include the source.)
Participants should not use any bot voting services, do not engage in vote buying.
The participation schedule is between Monday, October 14 , 2024 at 00:00 UTC to Sunday, - October 20, 2024 at 23:59 UTC.
Community moderators would leave quality ratings of your articles and likely upvotes.
The publication can be in any language.
Plagiarism and use of AI is prohibited.
Participants must appropriately follow #club5050 or #club75 or #club100.
Use the tags #dynamicdevs-s20w6 , #country (example- #tunisia, #Nigeria) #steemexclusive.
Use the #burnsteem25 tag only if you have set the 25% payee to @null.
Post the link to your entry in the comments section of this contest post. (very important).
Invite at least 3 friends to participate in this contest.
Strive to leave valuable feedback on other people's entries.
Share your post on Twitter and drop the link as a comment on your post.
Your article must get at least 10 upvotes and 5 valid comments to count as valid in the contest, so be sure to interact with other users' entries
Rewards
SC01/SC02 would be checking on the entire 16 participating Teaching Teams and Challengers and upvoting outstanding content. Upvote is not guaranteed for all articles. Kindly take note.
At the end of the week, we would nominate the top 5 users who had performed well in the contest and would be eligible for votes from SC01/SC02.
Important Notice: The selection of the five should be based solely on the quality of their post. Number of comments is no longer a factor to be considered.
Best Regards,
Dynamic Devs Team
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia