SEC S20W4|| Introduction to PHP Part -2
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
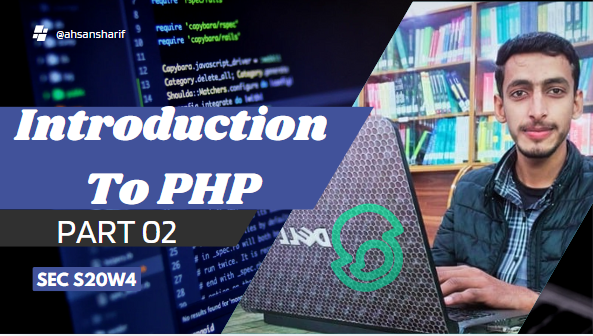
Made in Canva
Section 01
Explain in detail what exceptions are, what they are used for, and how they handle errors in PHP. Include an example of using the try-and-catch blocks. |
---|
In PHP, the way to handle errors that occur during the execution of a program is called an exception. Exceptions allow you to catch and handle errors in a more controlled and elegant way.
An interruption to the normal flow of script execution is called an exception. PHP throws an exception when something goes wrong. These allow exceptions to handle these problems systematically without destroying the entire script.
Why Use?
- Exceptions give developers control over how errors are handled. Instead of stopping the script, exceptions allow specific actions to be taken when an error occurs.
- The error handling logic is separated from the central court. refers to the flow of the block within the try statement. While the catch block handles errors.
- You can define different actions based on error and exception.
How do exceptions work in PHP?
When an error occurs, the normal flow of code execution is interrupted. At this point, PHP looks for an exception handler. This exception handler is usually written using a try-and-catch block.
Try block:
Here is the code that might be the exception PHP tries to execute inside the code.
Catch block:
If a small exception is filled in the try block, it is caught by the catch block. The catch block will handle the exception and determine what to do next.
Throw statement:
A throw statement is used to manually trigger an exception when an error condition is met.
Example:
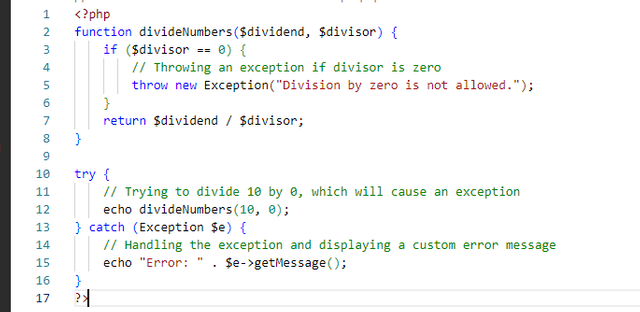
Describe six common types of errors in PHP, explaining each with concrete examples. |
---|
Parse Error:
This error appears when PHP doesn't understand your code. Because some things are missing like your syntax is wrong. In which you miss semi-colons or cancel parentheses and braces.
This example misses the semicolon that should have come after the echo statement. PHP doesn't understand your code correctly and it throws a syntax error.
Example:

Fatal Error:
A fatal error occurs when PHP encounters a problem that prevents it from executing a script, often when we try to use a function or class that doesn't exist.
Example:

Warning Error:
These errors are less severe than fatal errors, which occur when there is a problem with the script. But it allows PHP to continue other code. A warning error does not stop script execution.
Example:

Notice Error:
Notice Errors indicate minor problems such as attempting to access a variable that is not defined. These are not critical errors and do not prevent PHP from executing the script.
Example:

Deprecated Error:
A deprecated error occurs when a feature in PHP is marked as deprecated or will be removed in a future version. This will trigger an error that advises developers to update their code.
Example:
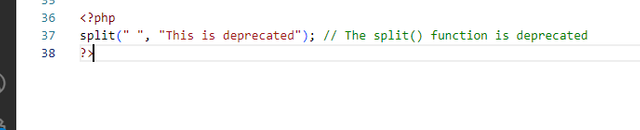
Notice of Undefined Error:
This error occurs when trying to access an array. PHP issues a notice but also continues executing on the script.
Example:
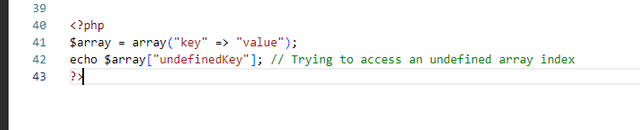
Explain what errors are in programming, with three personal screenshots of errors you have encountered, and describe each. |
---|
Program errors that prevent a program from working as expected These errors can occur for a variety of reasons. Like a syntax mistake or we get the logic wrong. Thus our output is generated incorrectly or behaves unpredictably. There are different types of errors in programming.
Syntax Error:
This error occurs when we do not fully follow the grammar of the programming language.

As we know such errors occur when we do not use proper programming grammar. As here the statement written in front of the echo is not preceded by a semicolon, it is an error.
Runtime Error:
Such errors occur during program execution, usually due to incorrect operations such as dividing by zero or attempting to access unavailable files.

In this example, the function is incorrectly called, so calling functions that do not exit returns an error.
Logical Error:
Here the program runs flawlessly without any syntax and runtime errors but generates false extremes because the logic is flawed.

The correct way to calculate an average is to write it in parentheses and divide by two.
Explain the include syntax in PHP and why it is important for reusing code, giving examples. |
---|
The include statement in PHP is used when the content of a PHP file needs to be inserted into another PHP file. This allows reusing code from multiple pages. It also helps us maintain the code efficiently by checking the contents of PHP's include file when you use include. and will execute as if written directly into the main script.
Syntax: include 'filename.php';
How it works?
Inserting Code:
When PHP encounters an include, its purpose is to read the specified file and add it to the script at the point where we need that content.
Continuing Execution:
If the file that is added has errors or is incomplete, PHP shows a warning but continues to execute the rest of the script.
Benefits:
Avoid Duplication:
You can create any content once and then reuse it anywhere on other pages. By reducing redundancy. We use the header footer and Navebar in different places.
Simplify Updates:
If you have a commonly used file that has been added to other pages, it needs to be changed. You will only need to make changes to one file. The rest of the file will automatically have the change wherever it is attached.
Improve Organization:
Code that is too large should be split into smaller files to improve code organization and readability. such as separating the layout from the logic.
Modularize Code:
You can divide a complex script into smaller parts keep each part in a separate file and add them as needed.
Example:
Here is the included code that is the header file: header.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Website</title>
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
Here is the main file where the header file is included. index.php
<?php include 'header.php'; ?>
<main>
<p>This is the main content of the home page.</p>
</main>
</body>
</html>
Describe the steps to connect a database to a PHP file, with code examples (connection variables, mysqli_connect(), or PDO). |
---|
I will first use the Xampp server to connect the PHP file to the database. In which I will start Apache and MySQL. And then I'll open the admin in front of MySQL where I'll create my database.
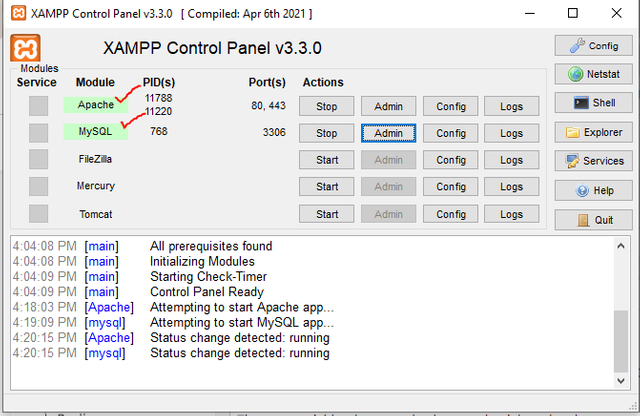
Create a Database:
Before we connect to the database, make sure you have created a MySQL database where all your data will be stored.
Define Connection Variable:
These are variables that store database credentials such as hostname, username, password, and database name.
Establish the Connection:
Now we have to use mysqli_connect() to establish a connection to the database.
Check for Connection Error:
Here, ensure that your connection to the database is successful. And handle errors appropriately.
Close the Connection:
Close your database connection to free up resources after running queries.
Code Connection:
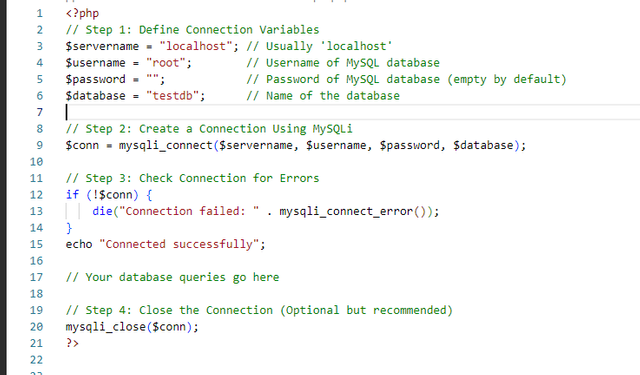
$servername:
This is the server where the database is hosted. This is usually localhost if you are working on a local environment.
$username:
This is the username for the MySQL database and is often the root of the local environment.
$password:
This is the password for the MySQL database and can often be left blank for local setups.
$database:
This is the name of the database you are connecting to.
Section 02
Create a PHP page (learn.php) and use the included syntax to import the header, navigation bar, sidebar, and footer. |
---|
To create a learn.php
page we will use the include statement to connect different pages to the learn.php
page, so first of all we need PHP files We will create the Header Footer Navebar Sidebar
files.
I have kept all these files in the include folder so that they can be separated and the files are as follows.
Header File:
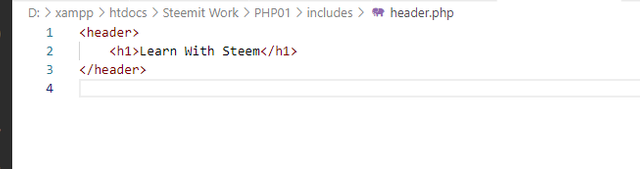
Navbar File:
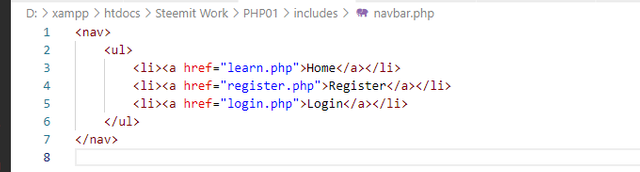
Sidebar File:
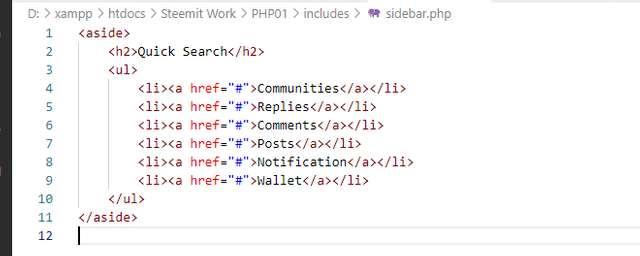
Footer File:
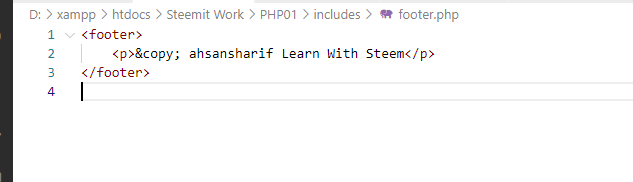
Learn File:
Now we need a main file in which we have to combine all these files using include. If you want to create your beautiful web page then the PHP code for the learn.php
file is as follows.
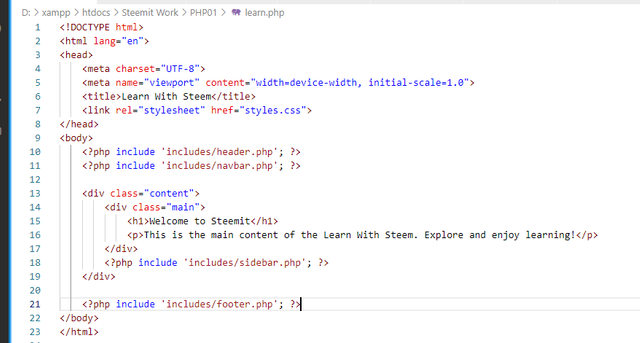
CSS File:
CSS is used to design all pages. With the help of this, they have been given everything like font size background color text color, etc. That CSS code is as follows.
![]() | ![]() |
---|
Final Output
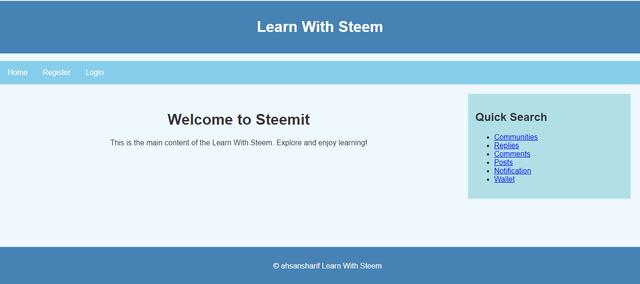
Create a database capable of storing the data for a registration page. |
---|
First I will use the XAMPP server to create the database. First, we will start Apache and MySQL on the XAMPP server. Then after that Admin is written in front of MySQL, and we have to open it, with the help of which we will have PHP My Admin main page open.
![]() | ![]() |
---|
Now the first thing to do is to create our database so for that we will write the name of the database and save it. Once the database is saved then we will add the table to it. In this table, our registration form data will be submitted and it will show here. For this table, we have to keep the first ID as primary and keep the rest as it is. With the help of this we have to create our table and database the following are the steps.
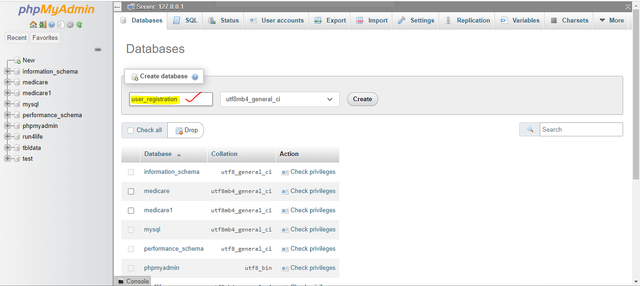
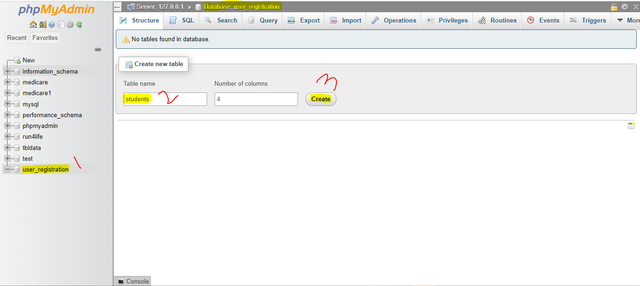
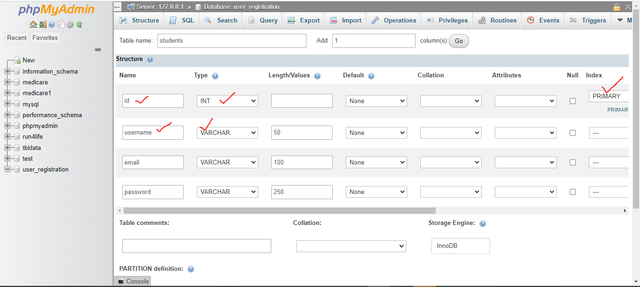
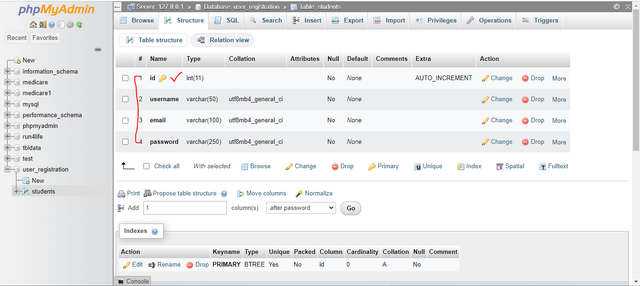
Create a PHP registration page to save the data of five students in a database. |
---|
We need a registration form to store student data. Through this registration form the student will submit his data and it will be saved in the database. For this first, we need to create a form. I created this form with the help of HTML and CSS and later scripted it in PHP.
Register File:
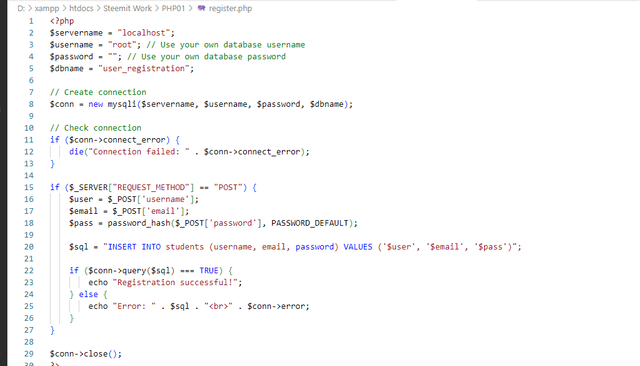
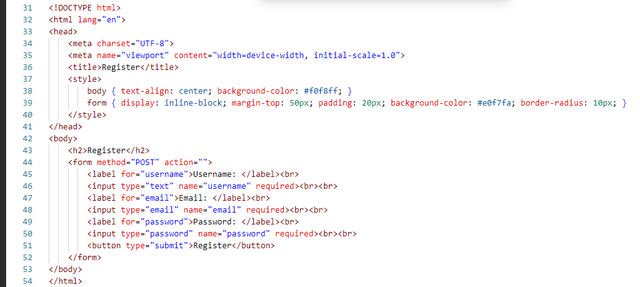
Form Output:
This is the final output of our form with the help of which we will enter the data from the user and it will be stored in the database. We have already created a table for the location in the database to be stored.
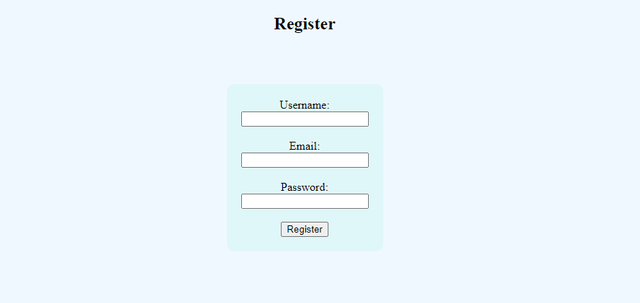
Data Store In Database:
Here you guys will be showing the data of five users which I have submitted with the help of the above form.
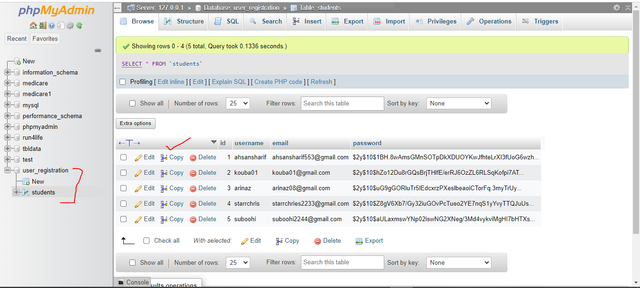
Video Output:
Create a PHP login page that verifies the login information with the ones stored in the database and redirects the user if successful. |
---|
First, we will create a login page with the help of which the user who is saved in the database will be able to login himself here and a successful message will be shown to him. For this, first, create a simple form with the help of HTML and CSS and then add PHP to it. And then with the help of the data stored in the database, we will log in the user.
Login File:
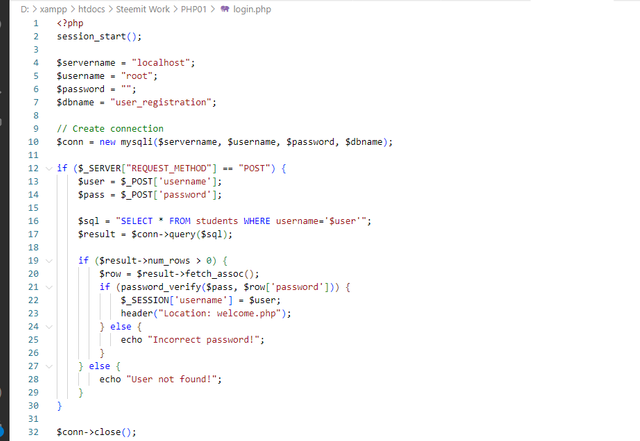
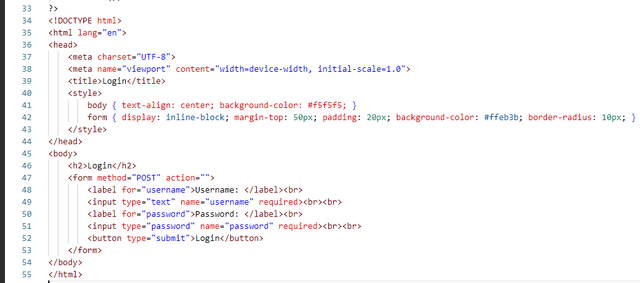
Login Form Output:
This is the final output of our login form. With this help, we will log in to our user from here.
![]() |
---|
Welcome Page:
This welcome message page will be shown after the user logs in.
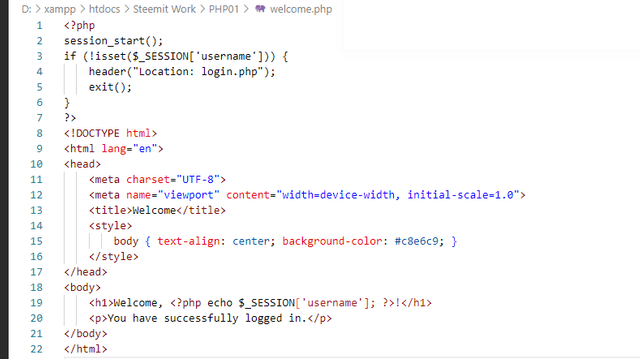
X:
https://x.com/AhsanGu58401302/status/1843009533696004318
@tipu curate
Upvoted 👌 (Mana: 0/7) Get profit votes with @tipU :)