Basic programming course: Lesson #6 Functions
Hello everyone! Today I am here to participate in the basic programming course by @alejos7ven. It is about learning the basics programming specifically functions. If you want to join then:
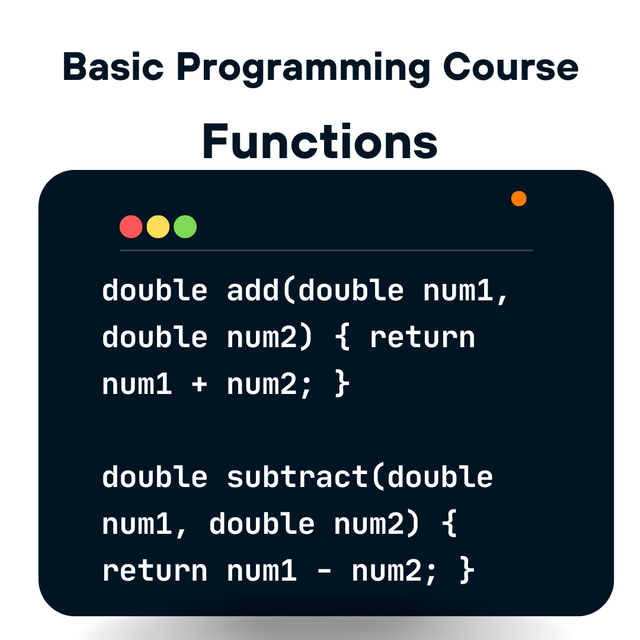.png)
Tell us about your experience with programming, was it your first time? Did you find it complicated? What was your favorite part of the course?
I am a student of Software engineering of final year. And my relation with programming is from the last 3 years. At first programming was looking very easy and like the child play but after some days I got to know that programming is also boring and it requires a lot of attention and creativity.
I learnt to tackle the problems of programming like understanding the complete syntax of the program and not to repeat syntax related mistakes. And if we talk about this recent programming course then I will say that it was a nice experience with this course.
This course helped me to revise my all the basic concepts and to implement them while solving the problems. Even I learnt new things about programming. Each task was very wonderful and it was really fascinating to me. Every time I attended the lecture it polished my skills and further enhanced them.
I efficiently learnt writing the algorithm of the program. It helps us to breakdown the bigger problems into simple problems and it tells us about the flow of the program. We can write the program keeping in view the algorithm of the program.
The one thing which I found technical and complicated for me to understand the code written by the professor because I am not familiar with Spanish and each time professor used to write the algorithms in Spanish compiler. Another complicated thing for me was to run the algorithm because it was only supported by PSeInt and i was again not familiar with this. I wrote algorithm in English but it gave errors and it did not run. So it was a complicated part of this course for me.
The favourite part of the course was to understand breaking down the complex problems in to simple parts and then resolving them. I really liked how we can simplify the complex problems by writing the Algorithm of the problems. And in this way we can solve complex problems in an efficient way. It also helps us to find different ways to solve the problems. And we can choose a simple and short way to solve that problem. It will save our time and efforts. Moreover lightweight code also is easy to compile and run it does not require more time space.
Add the functions to multiply and divide to the course calculator. Explain how you did it
In order to add multiplication and division functions to the course calculator I will show the basic code structure. Then I will show that how we can add multiplication and division functions in the calculator.
Steps to Add Multiplication and Division Functions
- I will start with the basic structure of a calculator. That basic calculator has addition and subtraction as an example.
- I will create two new functions for multiplication and division.
- I will ensure input validation for division to handle division by zero.
- Then I will change the main menu to include options for multiplication and division.
#include <iostream>
using namespace std;
// Function prototypes
double add(double num1, double num2);
double subtract(double num1, double num2);
double multiply(double num1, double num2);
double divide(double num1, double num2);
int main() {
double num1, num2;
int choice;
do {
// Display menu
cout << "Simple Calculator" << endl;
cout << "1. Add" << endl;
cout << "2. Subtract" << endl;
cout << "3. Multiply" << endl;
cout << "4. Divide" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
if (choice >= 1 && choice <= 4) {
// Get numbers from the user
cout << "Enter two numbers: ";
cin >> num1 >> num2;
}
switch (choice) {
case 1:
cout << "Result: " << add(num1, num2) << endl;
break;
case 2:
cout << "Result: " << subtract(num1, num2) << endl;
break;
case 3:
cout << "Result: " << multiply(num1, num2) << endl;
break;
case 4:
if (num2 == 0) {
cout << "Error: Division by zero is not allowed." << endl;
} else {
cout << "Result: " << divide(num1, num2) << endl;
}
break;
case 5:
cout << "Exiting..." << endl;
break;
default:
cout << "Invalid choice, please try again." << endl;
}
} while (choice != 5);
return 0;
}
// Function definitions
double add(double num1, double num2) {
return num1 + num2;
}
double subtract(double num1, double num2) {
return num1 - num2;
}
double multiply(double num1, double num2) {
return num1 * num2;
}
double divide(double num1, double num2) {
return num1 / num2;
}
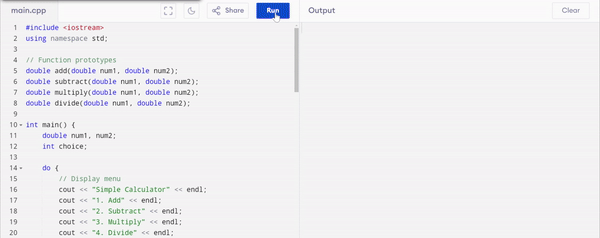
Here is a breakdown of how the above C++ calculator code works. I have included all the functions and steps in this working.
Program Structure
- The program defines four basic arithmetic functions. These are
add()
,subtract()
,multiply()
, anddivide()
. - In the
main()
function there is a loop. This loop continuously displays a menu to the user until they choose to exit by selecting option 5.
Displaying the Menu
At the start of the loop the program prints a menu with five options:
cout << "Simple Calculator" << endl;
cout << "1. Add" << endl;
cout << "2. Subtract" << endl;
cout << "3. Multiply" << endl;
cout << "4. Divide" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
- The user enters a number between 1 and 5. It is entered to select the operation they want to perform.
- If the user enters a valid option from 1 to 4 then the program asks them to input two numbers. Example is given below:
cout << "Enter two numbers: ";
cin >> num1 >> num2;
Switch-Case for Operation Selection
The program uses a switch
statement to handle the selection of the user.
a) Addition
If the user selects option 1
then the program calls the add()
function.
case 1:
cout << "Result: " << add(num1, num2) << endl;
break;
- The
add()
function returns the sum ofnum1
andnum2
:
double add(double num1, double num2) {
return num1 + num2;
}
b) Subtraction
If the user selects option 2
then the program calls the subtract()
function.
case 2:
cout << "Result: " << subtract(num1, num2) << endl;
break;
- The
subtract()
function returns the difference betweennum1
andnum2
.
double subtract(double num1, double num2) {
return num1 - num2;
}
c) Multiplication
If the user selects option 3
then the multiply()
function is called.
case 3:
cout << "Result: " << multiply(num1, num2) << endl;
break;
- The
multiply()
function returns the product ofnum1
andnum2
.
double multiply(double num1, double num2) {
return num1 * num2;
}
d) Division
If the user selects option 4
then the program calls the divide()
function. It has a check condition in which it checks division by zero.
case 4:
if (num2 == 0) {
cout << "Error: Division by zero is not allowed." << endl;
} else {
cout << "Result: " << divide(num1, num2) << endl;
}
break;
- If the divisor
num2
is zero then the program prints an error message and skips the division. - If the divisor is not zero then the
divide()
function returns the quotient.
double divide(double num1, double num2) {
return num1 / num2;
}
e) Exit Option
If the user selects option 5
then the program prints a message and exits the loop.
case 5:
cout << "Exiting..." << endl;
break;
Repeat Until User Exits
The do-while
loop ensures that the program keeps showing the menu until the user selects option 5
to exit.
} while (choice != 5);
- After each operation the program will return to the menu for the user to perform another calculation.
Handling Invalid Input
If the user enters an invalid option such a number not between 1 and 5 then the program will print a message and show the menu again.
default:
cout << "Invalid choice, please try again." << endl;
How Each Function Works
- Addition
add()
Function: It takes two numbers. It adds them and returns the result. - Subtraction
subtract()
: It takes two numbers. It subtracts the second from the first and returns the result. - Multiplication
multiply()
: It takes two numbers. It multiplies them and returns the result. - Division
divide()
: It takes two numbers. It divides the first by the second and returns the result. It checks if the divisor is zero before performing the division.
Example Run
First Run (Addition)
Simple Calculator
1. Add
2. Subtract
3. Multiply
4. Divide
5. Exit
Enter your choice: 1
Enter two numbers: 10 and 5
Result: 15
Second Run (Division with Zero):
Simple Calculator
1. Add
2. Subtract
3. Multiply
4. Divide
5. Exit
Enter your choice: 4
Enter two numbers: 10 and 0
Error: Division by zero is not allowed.
Third Run (Exit):
Simple Calculator
1. Add
2. Subtract
3. Multiply
4. Divide
5. Exit
Enter your choice: 5
Exiting...
This calculator program is simple but demonstrates the basic structure of input and output operations, conditional statements and function.
FINAL PROJECT: Create an ATM simulator. It must initially request an access pin to the user, this pin is 12345678. Once the pin is successfully validated, it must show a menu that allows: 1. view balance, 2. deposit money, 3. withdraw money, 4. exit. The cashier should not allow more balance to be withdrawn than is possessed. Use all the structures you learned, and functions.
First of all I want to ask the professor being a student that why most of the time an ATM program is given to the students. Because my teacher in the university also gave us this program to build an ATM machine. Maybe I can get an answer here from @alejos7ven. So moving next to build an ATM machine I will follow the following algorithm and criteria to build it.
But again before moving next I want to quote that ATM stands for Automated Teller Machine. Because many people uses on a daily basis ATM machine but they are not familiar about its stand for.
First if all I want to explain the simple algorithm of this ATM machine program that how it will work and perform different actions by giving access to the user while entering the correct pin.
- Start
- Setting the correct PIN which is
12345678
. I will set initial balance to1000
. - Then I will ask the user to enter their PIN.
- If the PIN is correct the program will proceed to the ATM menu.
- If the PIN is incorrect it will display an error and exit.
- Show the ATM menu
- 1.View Balance
- 2.Deposit Money
- 3.Withdraw Money
- 4.Exit
- Repeat until the user chooses to exit:
- If the user selects View Balance the the program will show the current balance.
- If the user selects Deposit Money then the program will show add the deposit amount to the balance.
- If the user selects Withdraw Money then the program will proceed to subtract the amount if sufficient balance exists. Otherwise it will show an error.
- If the user selects Exit then the program will end.
- End.
C++ Code for ATM Simulator
#include <iostream>
using namespace std;
// Function prototypes
bool validatePin(int enteredPin);
void showMenu();
void viewBalance(double balance);
double deposit(double balance);
double withdraw(double balance);
int main() {
const int PIN = 12345678; // Predefined PIN
int enteredPin;
double balance = 1000.00; // Initial balance
int option;
// Step 1: Validate the user PIN
cout << "Welcome to the ATM!" << endl;
cout << "Please enter your PIN: ";
cin >> enteredPin;
// Validate the entered PIN
if (!validatePin(enteredPin)) {
cout << "Incorrect PIN. Access Denied." << endl;
return 0; // Exit if PIN is incorrect
}
// Step 2: Show the ATM menu
do {
showMenu();
cin >> option;
switch(option) {
case 1:
viewBalance(balance);
break;
case 2:
balance = deposit(balance);
break;
case 3:
balance = withdraw(balance);
break;
case 4:
cout << "Thank you for using the ATM. Goodbye!" << endl;
break;
default:
cout << "Invalid option. Please try again." << endl;
}
} while (option != 4);
return 0;
}
// Function to validate the entered PIN
bool validatePin(int enteredPin) {
const int correctPin = 12345678;
return enteredPin == correctPin;
}
// Function to display the ATM menu
void showMenu() {
cout << "\nATM Menu" << endl;
cout << "1. View Balance" << endl;
cout << "2. Deposit Money" << endl;
cout << "3. Withdraw Money" << endl;
cout << "4. Exit" << endl;
cout << "Enter your option: ";
}
// Function to display the current balance
void viewBalance(double balance) {
cout << "Your current balance is: $" << balance << endl;
}
// Function to deposit money into the account
double deposit(double balance) {
double depositAmount;
cout << "Enter the amount to deposit: $";
cin >> depositAmount;
// Ensure valid deposit amount
if (depositAmount > 0) {
balance += depositAmount;
cout << "$" << depositAmount << " deposited successfully." << endl;
cout << "Your new balance is: $" << balance << endl;
} else {
cout << "Invalid deposit amount. Please try again." << endl;
}
return balance;
}
// Function to withdraw money from the account
double withdraw(double balance) {
double withdrawAmount;
cout << "Enter the amount to withdraw: $";
cin >> withdrawAmount;
// Check if the withdrawal amount is valid
if (withdrawAmount > balance) {
cout << "Insufficient funds. You cannot withdraw more than your current balance." << endl;
} else if (withdrawAmount <= 0) {
cout << "Invalid withdrawal amount. Please enter a positive number." << endl;
} else {
balance -= withdrawAmount;
cout << "$" << withdrawAmount << " withdrawn successfully." << endl;
cout << "Your new balance is: $" << balance << endl;
}
return balance;
}
Explanation of the Code:
PIN Validation:
- The correct PIN is set as
12345678
. - The user is asked to enter their PIN. The program checks if the entered PIN matches the correct one using the
validatePin()
function. - If the PIN is incorrect the program exits by denying access to the ATM.
- The correct PIN is set as
ATM Menu:
- Once the PIN is validated the program enters a
do-while
loop that continuously shows the ATM menu until the user chooses to exit. - The menu options include:
- Viewing the balance
- Depositing money
- Withdrawing money
- Exiting the program
- Once the PIN is validated the program enters a
View Balance:
- The
viewBalance()
function simply prints the current balance to the user.
- The
Deposit Money:
- The
deposit()
function asks the user for the amount to deposit. - The balance is updated only if the deposit amount is positive. If a negative or zero value is entered then an error message is shown.
- The
Withdraw Money:
- The
withdraw()
function asks the user for the amount they want to withdraw. - If the user tries to withdraw more than their current balance then the program will show an error message and prevent the transaction.
- The balance is updated only if the withdrawal amount is positive and within the current balance.
- The
Exit Option:
- Option 4 exits the program and thanks the user for using the ATM.
This ATM simulator uses conditionals, loops, functions, input and output handling and basic user interaction. The program manages the balance of the user and prevents invalid transactions like withdrawing more than the available balance or depositing invalid amounts.
Here is the video output to explain all the working of the above ATM Simulator. I have shown all the valid as well as invalid tasks to check the behaviour of the program practically. When I entered invalid pin then the program did not give me access to its further menu and operations and the program was exit. You can see I gave invalid deposit amount and it threw an error. Then when I tried to withdraw money more than the avail money it again threw an error that there are not sufficient funds.
X Promotion: https://x.com/stylishtiger3/status/1846463352286945645
Thank you so much @chant