Basic programming course: Lesson #6 Functions.
Hi friends,
I am Jyoti from India. I am happy to participate in the SEC S20 W6 contest:https://steemit.com/devwithseven/@alejos7ven/basic-programming-course-lesson-6-functions-esp-eng organised by @alejos7ven
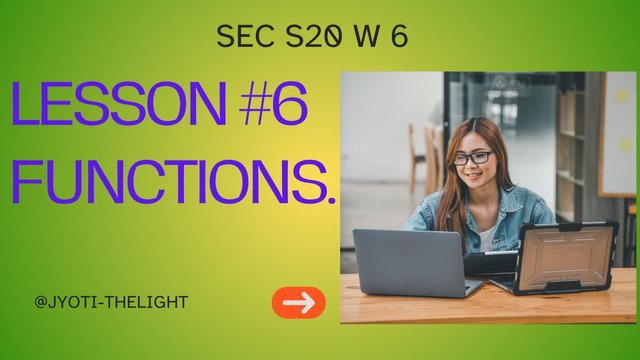.jpg)
Lesson #6 Functions. |
---|
Tell us about your experience with programming, was it your first time? Did you find it complicated? What was your favorite part of the course?
Programming is one of my favorites as I have already learned C and C Plus language. I am very happy that I participated in this particular course especially on Steemit organised by @alejos7ven. This gave me an opportunity to bring back my old memories. Also since I quit coding, this gives me an opportunity to take it up again. This six week course was very good for me and I participated in it very interestingly.
My favorite part of the six-week course was last week's course : "Lesson #5 Control structures. Part 2. Cycles". Because I got full marks and was awarded Excellent by the professor, which made me very proud and happy.

Add the functions to multiply and divide to the course calculator. Explain how you did it.
I'll add multiplication and division functions to the calculator program in C and explain the process step by step. Here's the updated code with the new functions:
#include <stdio.h>
// Function prototypes
float add(float a, float b);
float subtract(float a, float b);
float multiply(float a, float b);
float divide(float a, float b);
int main() {
float num1, num2, result;
char operator;
printf("Enter first number: ");
scanf("%f", &num1);
printf("Enter an operator (+, -, *, /): ");
scanf(" %c", &operator);
printf("Enter second number: ");
scanf("%f", &num2);
switch(operator) {
case '+':
result = add(num1, num2);
break;
case '-':
result = subtract(num1, num2);
break;
case '*':
result = multiply(num1, num2);
break;
case '/':
result = divide(num1, num2);
break;
default:
printf("Error: Invalid operator\n");
return 1;
}
printf("Result: %.2f\n", result);
return 0;
}
float add(float a, float b) {
return a + b;
}
float subtract(float a, float b) {
return a - b;
}
float multiply(float a, float b) {
return a * b;
}
float divide(float a, float b) {
if (b != 0) {
return a / b;
} else {
printf("Error: Division by zero\n");
return 0;
}
}
Now, let me explain how I added the multiplication and division functions to the calculator:
Function Prototypes: I added two new function prototypes at the beginning of the program:
float multiply(float a, float b);
float divide(float a, float b);
These prototypes declare the functions before they are used, allowing the compiler to know their return types and parameters.
Switch Statement: In the main() function, I added two new cases to the switch statement:
case '*':
result = multiply(num1, num2);
break;
case '/':
result = divide(num1, num2);
break;
These cases handle the multiplication and division operations when the user enters '*' or '/' as the operator.
Multiplication Function: I implemented the multiply() function:
float multiply(float a, float b) {
return a * b;
}
This function simply returns the product of the two input numbers.
Division Function: I implemented the divide() function:
float divide(float a, float b) {
if (b != 0) {
return a / b;
} else {
printf("Error: Division by zero\n");
return 0;
}
}
This function checks if the divisor (b) is not zero before performing the division. If it is zero, it prints an error message and returns 0 to avoid a division by zero error.
User Input: I updated the prompt for the operator to include '*' and '/' as options:
printf("Enter an operator (+, -, *, /): ");
By adding these new functions and updating the switch statement, the calculator can now handle multiplication and division operations in addition to addition and subtraction. The program structure remains the same, maintaining modularity and readability while expanding its functionality.

FINAL PROJECT: Create an ATM simulator. It must initially request an access pin to the user, this pin is 12345678. Once the pin is successfully validated, it must show a menu that allows: 1. view balance, 2. deposit money, 3. withdraw money, 4. exit. The cashier should not allow more balance to be withdrawn than is possessed. Use all the structures you learned and functions.
I used to make this program in C++, and this program demonstrates the use of functions also I used loops, conditional statements, and basic input/output operations in C++. It also includes some error handling to make the simulator more robust. Here is the Code:
#include <iostream>
#include <iomanip>
#include <limits>
const int PIN = 12345678;
double balance = 1000.0; // Initial balance
void clearInput() {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
bool validatePin() {
int enteredPin;
std::cout << "Enter your PIN: ";
std::cin >> enteredPin;
return enteredPin == PIN;
}
void viewBalance() {
std::cout << "Your current balance is: Rs" << std::fixed << std::setprecision(2) << balance << std::endl;
}
void deposit() {
double amount;
std::cout << "Enter the amount to deposit: Rs";
std::cin >> amount;
if (amount > 0) {
balance += amount;
std::cout << "Deposit successful. New balance: Rs" << std::fixed << std::setprecision(2) << balance << std::endl;
} else {
std::cout << "Invalid amount. Deposit cancelled." << std::endl;
}
}
void withdraw() {
double amount;
std::cout << "Enter the amount to withdraw: Rs";
std::cin >> amount;
if (amount > 0 && amount <= balance) {
balance -= amount;
std::cout << "Withdrawal successful. New balance: Rs" << std::fixed << std::setprecision(2) << balance << std::endl;
} else if (amount > balance) {
std::cout << "Insufficient funds. Withdrawal cancelled." << std::endl;
} else {
std::cout << "Invalid amount. Withdrawal cancelled." << std::endl;
}
}
void showMenu() {
std::cout << "\nATM Menu:" << std::endl;
std::cout << "1. View Balance" << std::endl;
std::cout << "2. Deposit Money" << std::endl;
std::cout << "3. Withdraw Money" << std::endl;
std::cout << "4. Exit" << std::endl;
std::cout << "Enter your choice (1-4): ";
}
int main() {
std::cout << "Welcome to the ATM Simulator!" << std::endl;
if (!validatePin()) {
std::cout << "Invalid PIN. Access denied." << std::endl;
return 1;
}
std::cout << "Access granted." << std::endl;
int choice;
do {
showMenu();
std::cin >> choice;
switch (choice) {
case 1:
viewBalance();
break;
case 2:
deposit();
break;
case 3:
withdraw();
break;
case 4:
std::cout << "Thank you for using the ATM. Goodbye!" << std::endl;
break;
default:
std::cout << "Invalid choice. Please try again." << std::endl;
clearInput();
}
} while (choice != 4);
return 0;
}
This ATM simulator includes the following features:
PIN validation (12345678)
Menu with options to view balance, deposit money, withdraw money, and exit
Balance checking to prevent overdrawing
Input validation for deposits and withdrawals
Use of functions to organize the code
Proper use of loops and conditional statements
The program starts by asking for a PIN. If the correct PIN is entered, it grants access to the ATM menu. The user can then choose from the available options until they decide to exit.
The clearInput() function is used to clear any invalid input to prevent infinite loops in case of incorrect input types.The balance is initialized to Rs.1000.0 for demonstration purposes. You can modify this initial balance as needed.
Lets compile this code :
I am using an online C++ compiler to compile this program.
After typing the code of the program I made it and hit run then the compiler asked to enter the PIN
After entering the pin number the compiler gives us four options and asks us to select one of them
I select the second option deposit and after pressing the 2 number it asks the number of deposit amount we have to make.
Here I entered Rs. 5000 and it gives the result as deposit successful and the balance amount Rs. 6000, it asks again to choose any option to proceed
I entered 3 to withdraw the amount.
It asks to enter the amount to withdraw, I entered Rs 2000 and it shows a balance of Rs 4000, these the code was successful.

I would like to invite
to take part in this contest.
Discord : @jyoti-thelight#6650 Telegram :- https://telegram.org/dl
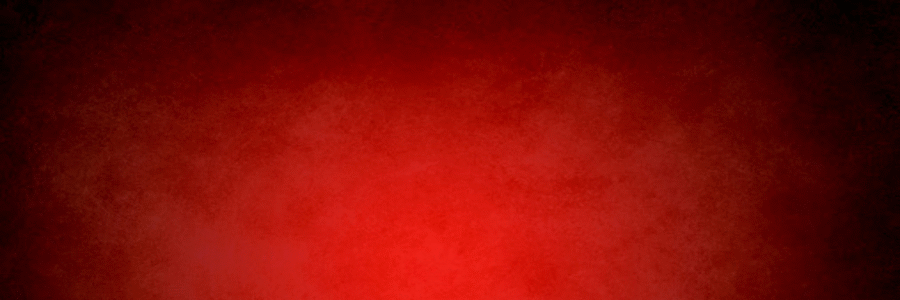
Upvoted. Thank You for sending some of your rewards to @null. It will make Steem stronger.
Thank you @malikusman1 for the valuable support
You have written really well mam, i must confess that i enjoyed reading through your blog.
This course have really educated me about programming and what i am going to face in the university.
Thanks to the steemit team for providing us with this wonderful opportunity.
I will try my best to participate.
I wish you success and do have a wonderful day 😊
Hola buenas noches amiga veo que realizaste una excelente participación dominando muy bien el tema tu participación es muy excelente
Le deseo mucha suerte en su participación